An End-to-End Guide on Angular Technology and Development
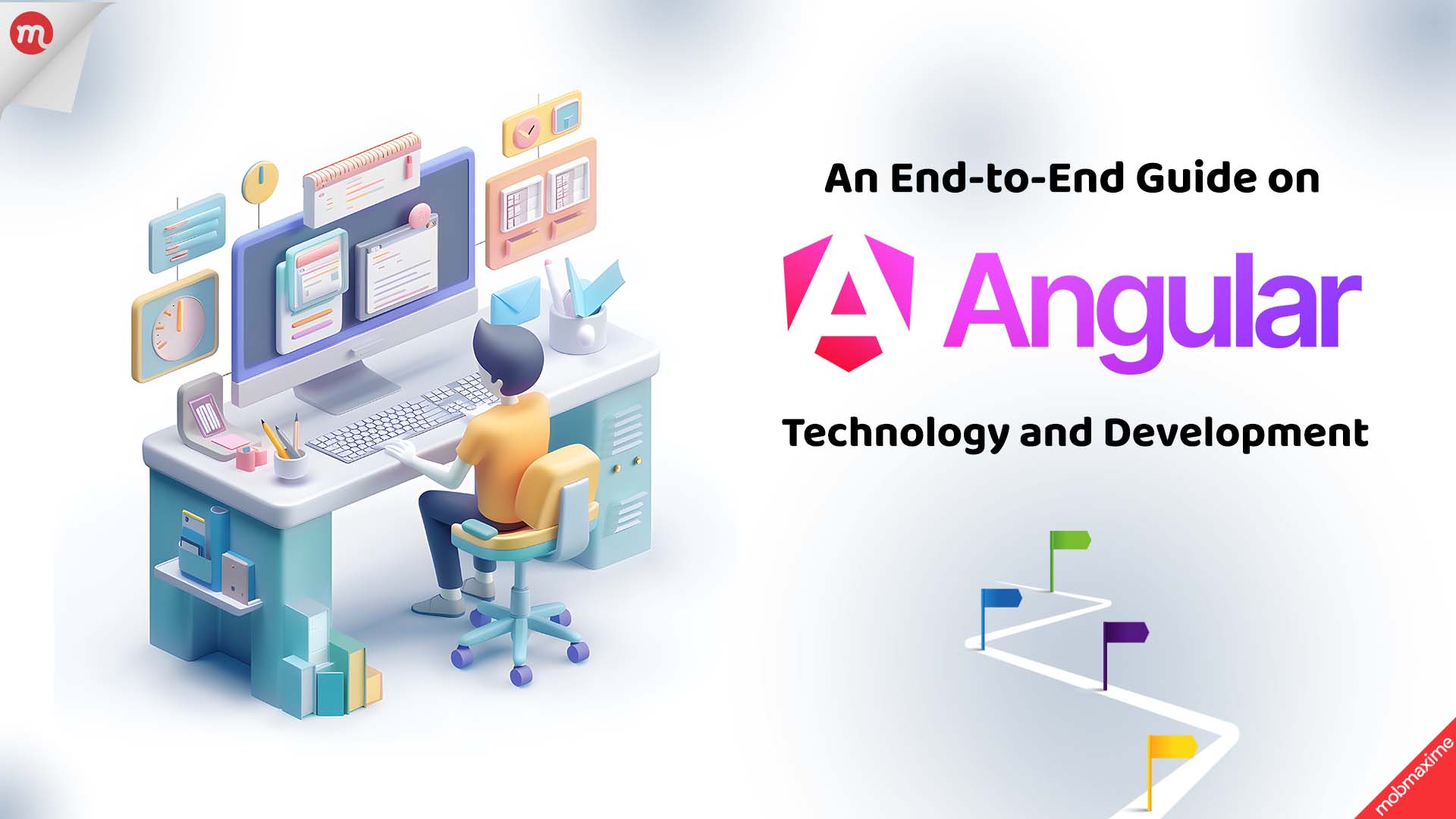
Angular is among the top web frameworks empowering developers of all levels to build reliable applications. A key technology for creating single-page applications (SPAs), Angular boasts a modern design and a rich ecosystem of tools and libraries. A vast ecosystem of pre-built UI components grants the technology with reactive data management while simplifying SPA development.
This web framework takes development ahead of traditional development modes and offers better functionality. Where features like reusability and easy maintainability are the cornerstones of development with Angular, it also has a Declarative UI that makes working with Angular and building SPAs. Furthermore, as a top Angular development company, we can assure you that working with Angular’s CLI and style guide makes everything much easier.
Traditional Web Development | Angular Web Development |
HTML and CSS Layout | Angular Components |
Static Content | Dynamic Content |
Server-Side Rendering | Client-Side Rendering |
Angular is maintained by Google, and it regularly brings forward a suite of developer-friendly tools, APIs, and libraries effectively streamlining the development work.
A Detailed Overview of Angular
// app.component.ts
import { Component } from ‘@angular/core’; @Component({ selector: ‘app-root’, templateUrl: ‘./app.component.html’, styleUrls: [‘./app.component.css’] }) export class AppComponent { title = ‘Welcome to Angular!’; } |
The small code snippet above has a component (AppComponent) attached with a property title. It also has a templateUrl property which specifies the HTML item connected with the component.
Moreover, within the template, you will see <h1> element with double curly braces. It’s used to show the value of title property. This code snippet is the representation of one-way data binding in Angular.
Prerequisites for Working with Angular
For working with Angular smoothly, developers need to be familiar with some of the basic concepts. A good understanding of the following will create a smooth working environment with Angular;
-
Core Web Technologies
HTML, CSS, and JavaScript are the core technologies, or we can say, building blocks of any web application. Similarly, if you want to build applications with Angular, an understanding of these three is important.
Through these, it will be easier for you to create web pages and style them. JavaScript is useful for adding dynamic functionality, which contributes to application performance and speed.
-
Tools for Angular
Angular applications are written in TypeScript because they offer static typing, through which it’s easier to detect errors during development. Plus, you will experience better code reliability and maintainability.
Another important tool is CLI, which provides benefits like code completion, type checking, and refactoring. Together with the tools and utilizing TypeScript, you can experience a smoother development experience wherein it’s easier to detect code anomalies and integrate with other Angular features.
Core Concepts To Understand for Angular Development
Angular’s architecture heavily relies on some fundamental concepts that drive the development process in the right direction.
-
Components
Components are also the building blocks of the applications you wish to build with Angular. These components help cover logic and the user interface part of the application. As a result, you will find it easier to manage application code and also reuse it when required.
Let me explain what I mean;
- Components in Angular define views which are the basic sets of screen elements that can be changed and modified according to the program logic.
- Components also define services that are responsible for running background functionality, which is not directly related to views.
- Components and services together are decorators responsible for providing metadata and instructing Angular on how to use them.
Angular components promote modularity in the development process while allowing you to work on complex user interfaces. This is where the Angular UI component library is useful and helps build self-contained components.
According to the structure and layout of Angular, each component has three parts;
- TypeScript class defining the behavior of the component.
- HTML template defining the component’s appearance.
- CSS for styling.
import { Component } from ‘@angular/core’; @Component({
selector: ‘app-example’,
template: ‘<button (click)=”onClick()”>Click me</button>’,
})
export class ExampleComponent {
onClick() {
console.log(‘Button clicked!’);
}
}
While we are at it, let me also talk about components in some detail. I will briefly talk about the things that you will come across when working with Angular;
- Component Lifecycle: Every component has a lifecycle in Angular, which begins with the instantiation of the component class, progressing to rendering the component view and its child views.
SourceFollowing the life cycle, developers can use lifecycle hooks to insert custom logic into the code. These hooks are;
Lifecycle Hook Description ngOnChanges() Used when an input property of the app directive changes. It received a SimpleChanges object for the current and previous property values. ngOnInit() This hook is called after the component’s initialization and is used only once. ngDoCheck() It’s called for changes in every detection run and is also used to identify plus act upon changes. ngAfterContentInit() It’s called after component content is initialized and is also used to access child components. ngAfterContentChecked() It’s called after checking of component content and is also used to access child components. ngAfterViewInIt() It’s called post-checking of component content and is also used to access child components. ngAfterViewChecked() It’s called after all the checks of a component view and is used to access child components. ngOnDestroy() It’s called before destroying the direction and is also used for cleanup tasks.
- View Encapsulation: With this, the component’s style in Angular can be encapsulated within the component element of the host. As a result, adding new styles won’t affect the rest of the application.
For this, we use the decorator component to run the encapsulation option and control how it is applied to the components.
- Component Interaction: This highlights the mechanism used in which Angular components within an application exchange data and collaborate together to complete an action. This interaction is pivotal for an application to perform dynamically and stay interconnected.
- Sending Data to a Child Component: The parent component passes data to the child component with the @Input() decorator. This command will mark a property or component in the parent component, which qualifies as input for the child component. As a result, changes in the input property in the Parent component will be seen in the Child component.
Parent Component import { Component } from ‘@angular/core’;
@Component({
selector: ‘app-parent’,
template: `
<h1>Welcome, {{ name }}!</h1>
<app-child [userName]=”name”></app-child>
`
})
export class ParentComponent {
name = ‘John Doe’;
}
Child Component
import { Component, Input } from ‘@angular/core’;
@Component({
selector: ‘app-child’,
template: `
<p>Hello, {{ userName }}!</p>
`
})
export class ChildComponent {
@Input() userName: string;
}
- Sending Data to a Child Component: The parent component passes data to the child component with the @Input() decorator. This command will mark a property or component in the parent component, which qualifies as input for the child component. As a result, changes in the input property in the Parent component will be seen in the Child component.
-
- Sending Data to Parent Component from Child: For this, we use the @Output() decorator command and the EventEmitter class. As a result, the parent component will listen to the event emitted by the Child component and work accordingly.
Child Component import { Component, Output, EventEmitter } from ‘@angular/core’;
@Component({
selector: ‘app-child’,
template: `
<button (click)=”greetParent()”>Greet Parent</button>
`
})
export class ChildComponent {
@Output() greet = new EventEmitter<string>();
greetParent() {
this.greet.emit(‘Hello from the Child!’);
}
}
Parent Component
import { Component } from ‘@angular/core’;
@Component({
selector: ‘app-parent’,
template: `
<app-child (greet)=”onGreet($event)”></app-child>
<p>Message from Child: {{ message }}</p>
`
})
export class ParentComponent {
message = ”;
onGreet(message: string) {
this.message = message;
}
}
- Sending Data to Parent Component from Child: For this, we use the @Output() decorator command and the EventEmitter class. As a result, the parent component will listen to the event emitted by the Child component and work accordingly.
- Content Projection: This is a pattern employed to insert the content into another component. Content projection can take three forms;
- Single Slot Content Projection: Component accepts content from a single source.
- Multi-Slot Content Projection: Component accept content from multiple sources.
- Conditional Content Projection: In this, the component uses a condition for content projection and accepts it only when the underlined conditions are met.
- Rendering Components Dynamically: Angular’s capability allows you to create and insert components into the app’s DOM at runtime. As one of the important Angular application modules, this does away with defining the components statically in the component templates. As a result, developers will experience better flexibility and modularity in Angular components.
- Custom Elements: These are the elements in Angular we use to create reusable HTML tags, irrespective of the framework. These HTML tags are used to encapsulate Angular components and enable you to package Angular components within custom HTML elements. As a result, the custom elements you build will gain interoperability, integrating seamlessly with frameworks and libraries.
-
Modules
Angular is a modular framework and its modularity is credited to the NgModule, popular for simplifying code organization. With NgModule, developers can create distinct modules, which can be loaded when required with another Angular feature (Lazy Loading).
The Angular application modules command is essential for configuring the injector compiler and helping with organizing the related components. In other, it’s a way to consolidate app features that belong together, but in discrete units.
As a result, you will end up creating a modular with granular control that is easily scalable. The NgModule command is used when consolidating app components, directives, and pipes into blocks representing functionality.
-
Templates
Templates in Angular represent a set of HTML defining the structure and appearance of a component’s view. In other words, it provides instructions for how every component is rendered on the screen.
While coding, you have to use standard HTML tags and attributes, combining them with the best Angular web templates, including;
Syntax Command Description Interpolation {{ }} Required to embed expressions into the template, including variables or calculations. Property Binding [ ] It’s used to set element property values, but dynamically. Event Binding ( ) A command we employ to respond to user events and trigger component methods. Structural Directives (nglf, ngFor) It’s used to add or remove elements from the DOM dynamically. Attribute Directives (ngClass, ngStyle, etc) It’s used to manipulate an element’s behavior or appearance. One thing to note here is that every angular component connected with a template is defined using the property templateUrl.
import { Component } from ‘@angular/core’; @Component({
selector: ‘app-my-component’,
templateUrl: ‘./my-component.component.html’,
})
export class MyComponent { }
- Text Interpolation: Text interpolation is one of the techniques we use to integrate expressions in markup directly. Plus, the added expressions undergo dynamic evaluation and the results are then displayed with rendered content.
An example of this property is a custom greeting displayed to the users, like their name, designation, etc. By default, interpolation uses double curly braces ({{ }}).
At rendering, Angular evaluates the expression you have added in the braces, replacing it with the resulting value. This value can be a variable, a component’s property, or even a function call. Lastly, template interpolation has a one-way binding mechanism. So the changes made to the expression value in the component will be updated in View, automatically.
- Template Statements: We have recently discussed binding targets; template statements are tasked with responding to these events raised by binding targets. This includes elements, components, or directives. You will find these within double quotes and on the right side of an event binding. These templates are often used in applications for engaging users through actions, like displaying dynamic content or submitting forms.
- Data Binding in Angular
Data binding is the process frameworks like Angular use to display data on View. Defining the communication between components and their view, data binding grants dynamism and interactivity to the applications.
Angular has four types of binding mechanisms;
-
- Property Binding: A one-way data binding mechanism allowing developers to HTML elements’ properties. This includes updating component property value and connecting that value to an HTML element in View. Using the [] syntax for data binding, this type of binding is often used for toggling functionality and sharing data between components.
- Attribute Binding: This form of binding allows you to connect an element’s HTML attributes in the template. Mostly used to dynamically update the element’s behavior or appearance, it’s applicable when the conditions for binding are the same.
- Class Binding: Use class binding to add or remove CSS classes from an element. Hence, its useful for applying styles subject to certain conditions.
- Style Binding: Another one-way data binding technique is applicable to set CSS property value on the element. However, for this, you must have a CSS property that you will bind to the element.
- Event Binding: Event binding represents the flow of information from View to Component, but only after the event is triggered. What qualifies as an event can be anything from a mouse click to a keypress, etc. From View, the data travels to the component with the updated information.
Did you realize something? Event binding is the exact opposite of property binding, in which data transfers from component to view.
-
- Two-Way Data Binding: In this form of binding mechanism, data flows from Component to View and back. This method of Angular data binding ensures that the component and view elements are synchronized and changes on either end show on both.
- Pipes
Pipes are used in template expressions for one purpose. They accept an input value and, in return, send a transformed value. These are valuable in Angular development services because you can utilize them throughout the application and declare each pipe only once.
Since these can be used only once, you will find built-in pipes within Angular, including;
-
- DatePipe
- UpperCasePipe
- LowerCasePipe
- CurrencyPipe
- DecimalPipe
- PercentPipe
- AsyncPipe
- JsonPipe
The purpose of adding these is to format the data value into the required format according to the defined function. For instance, a value-added with DatePipe will transform the value into a set format according to locale rules.
- Template Reference Variables
Template Reference variables help access all the properties of any element inside the DOM. It can call upon;
-
- DOM Element
- Directives
- Angular components
- Web components
To use any of these reference variables in development, you must first create the input element and then add the template reference to the input field using a # tag. Next, using the button click method, “changeTitle()” binds the template reference. Once done, you will have full access to the input field.
-
Directives
Directives are classes popularly used in Angular to add new behavior to DOM elements or even modify existing behavior. These directions represent markets on the DOM elements like attributes, element names, comments, or CSS classes. As the name suggests, the directives instruct the Angular compiler to attach the asked specific behavior to that element.
-
- Built-in Directives: In the Angular UI component library, you can find built-in directives allowing you to manipulate the DOM and add dynamic behavior to the applications. These built-in directives have two subtypes;
- Structural Directives: Directives that change the DOM structure by way of adding, removing, or manipulating elements are structural directions. Commands for structural directives include ngIf, nfFor, ngSwitch, and ngTemplateOutlet.
- Attribute Directives: When it’s about changing the appearance or behavior of an element, component, or another directive, you can use Attribute Directives. The commands for these are ngClass, ngStyle, ngModel.
- Directive Composition API: Directives offer a better way to encapsulate reusable behavior. From applying attributes to the application, it can also help with CSS classes and adding event listeners to an element. The purpose of using directive composition API is to apply them to a component’s host element; here comes the important bit from within the component TypeScript class.
- Built-in Directives: In the Angular UI component library, you can find built-in directives allowing you to manipulate the DOM and add dynamic behavior to the applications. These built-in directives have two subtypes;
Hence, you can build a more modular and reusable code while encapsulating complex behavior and reducing the amount of code required.
-
Services
Services in Angular represent reusable classes that can contain application logic, data, or functionality. Plus, you can reuse the encapsulated input with different components and directions or even with other services. This makes Services an important building block to create module Angular applications.
Any Service added to the Angular code gets instantiated once in an application. You can also say that these services have methods that maintain data for an application for its entire lifetime, ensuring that the data remains available all the time.
-
Dependency Injection
Angular dependency injection allows developers to provide dependencies to components, services, and other loosely coupled parts of the application. Being wired into the Angular framework, DI allows the compatible components to configure dependencies according to their requirements.
This brings me to two main concepts related to dependency injection in Angular: Dependency Consumer and Dependency Provider. The interaction between these two is managed by an abstraction called Injector.
Here’s how everything works;
- Dependency Request: An Angular component or service requests a dependency through its constructor arguments.
- Injector Lookup: The Injector, which is responsible for completing the dependency request, starts to search;
- The majority of the time, this is the injector associated with the component or service that’s making the request.
- Angular creates a hierarchy of injectors, including child components inheriting properties from parent injectors.
- Registry Check: The injector will check to ensure that an instance of the requested dependency already exists. If it does, the registry will hold the previously created instances.
- Instance Creation: If no instance is found, the injector will create a new instance. As a result, all the required dependencies of the new instance are resolved through further injector lookups.
- Instance Storage: The newly created dependency instances are stored in the injector’s directory for any future requests.
- Dependency Injector: The injector sends the resolved dependency instance into the component or service that has requested the same.
Going forward in our discussion on Angular Injector, let’s discuss a few related concepts;
- Create Injectable Service
Injectable service in Angular is a TypeScript class added with an @Injector()decorate to provide metadata, enabled dependency injection system for creating and managing service class instances.To create an Injectable service, use the code ng generate service my-new-service, and this will create a new file with the same name. To inject the service into the component,import { Component } from ‘@angular/core’; import { MyNewService } from ‘./my-new-service.service’;
@Component({
selector: ‘app-my-component’,
templateUrl: ‘./my-component.component.html’,
styleUrls: [‘./my-component.component.css’]
})
export class MyComponent {
data: string = ”;
constructor(private myNewService: MyNewService) { }
ngOnInit() {
this.data = this.myNewService.getData();
}
}
These injectables are fundamental in Angular application development as they provide a better way to share functionality, data, and state to the different parts of the application.
- Injection Context
While working with Angular dependency injection, you will come across injection context, which is a runtime context utilized when the current injector is present. However, these injectors will only work when the application code is executed in this context.
The DI system looks internally towards the runtime context to work with the available current injector. So this means that an injector can only work when the code is executed in this context.
- Hierarchical Injectors: It’s an important concept of DI, referring to the method injectors are organized in a tree-like structure parallel to an app’s component tree.
In an Angular application, every component can have its own injector. However, let me clear this out. Every component does not need an injector, so don’t focus on creating injectors for every component you add, regardless of the fact that it needs one or not.
Still, we try to add an injector wherever possible for every component. This means there are multiple injector instances operating at different levels of the component tree. Here’s a small graphical representation of the hierarchical injector.
Assuming the component at the bottom requests a dependency, Angular will attempt to satisfy that dependency with the provider’s registered component. However, if the component injector lacks a provider, the request will move to the parent component injector. This process will continue until Angular finds an injector that can satisfy the request until all component ancestors are checked, after which the users will be shown an error.
Explaining Auth Guards in Angular
Angular route guards or Auth Guards is a security mechanism we use in Angular development services to control access to specific routes. The rules for protection are based on the user’s authentication or authorization, which is also predefined.
Hence Auth Guards are essentially gatekeepers to intercept attempts by unauthorized actors. Using these, you can set Role-Based Access Control (RBAC), which means it’s easier to restrict access to the routes according to the user’s role, like admin, editor, user, etc.
Working Process of Auth Guards
- Route Guards and Navigation: The moment a user attempts to access a protected route, the Angular router activates the connected Auth Guard.
- Checking and Inspection: Using the CanActivate, which is a lifecycle method, the Auth Guard will return a boolean value or an observable to resolve the same, and this can lead to two instances;
-
- Returns True Value: With an authenticated or authorized user or when no authentication is required, the canActivate method will return true value. This means the navigation will proceed smoothly.
- Returns False Value: In case the user wanting access is not authorized and the Auth Guard determines the same, it will deny the request, and the canActivate() method will return false. This redirects the user back to the main page.
Implementation of Auth Guard
To implement Auth Guard, you must write the authorization and authentication logic inside the canActivate function. You have to add a simple command in the CLI;
ng g guard services/auth
This command will create an Auth Guard function in the Services folder, named auth.
import { Injectable } from “@angular/core”;
import { ActivatedRouteSnapshot, CanActivate, Router, RouterStateSnapshot, UrlTree } from “@angular/router”; import { AuthService } from “./auth.service”; @Injectable() export class AuthGuard implements CanActivate { constructor( private authService: AuthService, private router: Router) { } canActivate( route: ActivatedRouteSnapshot, state: RouterStateSnapshot): boolean | Promise<boolean> { var isAuthenticated = this.authService.getAuthStatus(); if (!isAuthenticated) { this.router.navigate([‘/login’]); } return isAuthenticated; } } |
The code excerpt above is used to add the Auth Guard mechanism to the Angular application. As we have discussed above, Auth Guard has to return a true value to allow access. In the code above, the getAuthStatus method is used for the same. If this value returns a true value, access is granted. If it returns a false value, access is denied.
HTTP Interceptors in Angular
A powerful and important feature of Angular, HTTP interceptors allow developers to intercept and modify HTTP requests. Well, one would ask why we need to intercept HTTP requests?
Well, there are many reasons…
- Centralized Control Over the Network of Communication: With interceptors, you can modify the outgoing HTTP requests before they reach the server. It’s useful to add authentication headers for securing communication. Moreover, you can also inject additional data into the request while transforming request data into a format that is expected by the server.
In addition, you can standardize request configuration through common request implementation in an interceptor. This is useful to ensure the application’s behavioral consistency. Doing so means you won’t have to repeat code for adding authentication and handling base URL tasks.
- Better Application Functionality and User Experience: Interceptors can trigger progress indicators or loading animations during the time requests are generated. As a result, the user experience tends to improve while bringing visual feedback on ongoing network activity. You can also use interceptors to implement caching strategies for HTTP requests.
What’s the benefit of this, you may ask? Well, caching implies reusable data is saved and presented the next time without rendering it from scratch. This reduces server load time, effectively application performance and user experience.
- Error Handling is Streamlined: With interceptors, you can build a single point of access to handle errors in HTTP requests. Even better, you can implement bespoke error-handling logic within the interceptor, including;
-
- Differentiating between different error types
- Customize error messages for the end users
- Log errors for debugging
- Include retry logic for failed requests
Ever since we started using HTTP interceptors, it has become easier to write robust and maintainable code, allowing us to build user-friendly applications.
Details on Angular Router / Angular Routing
Routing in Angular is a powerful feature, enabling developers to create single-page applications, especially ones that have multiple views. Each of these views corresponds to a different URL. Routing determines the way an application responds to a specific URL or path. The process involves mapping URLs to different application components or views and showing appropriate content.
Looking at it from the client’s side, routing allows them to switch or navigate between separate views or pages without having to load a new HTML page. It does so by dynamically updating the application or website content by loading necessary components and data.
Here, routing is managed by Angular routers through flexible and easy-to-use APIs, especially used for defining routes and handling navigation. Leveraging its routing and navigation system, Angular offers flexibility, enabling developers to build sophisticated applications. To implement this service, we use the @angular/router command, leading to navigation from one view to the next as users perform actions simultaneously.
- Router States: Angular router states represent the different arrangements of components executed during navigation to define what will be visible on the screen.
RouterStateSnapshot is a related element to Router States, representing an immutable data structure showing the state of the Router at a particular moment. In addition, there’s RouterState, which also represents a router’s state, but when it changes over time.interface RouterStateSnapshot { root: ActivatedRouteSnapshot;
}
interface ActivatedRouteSnapshot {
url: UrlSegment[];
params: {[name:string]:string};
data: {[name:string]:any};
queryParams: {[name:string]:string};
fragment: string;
root: ActivatedRouteSnapshot;
parent: ActivatedRouteSnapshot;
firstchild: ActivatedRouteSnapshot;
children: ActivatedRouteSnapshot[];
}
The above code represents a tree of activated route snapshots. All the nodes in this tree know about the “consumed” URL segments, the parameters extracted, and the resolved data.
In RouterStates, the path defines the URL pattern, which matches the state. The Component is the property displayed for the chosen state. Optional commands are redirectTo, used for sending users to a different route and Children, representing an array of child router states that help define nested routes in a parent state.
- Navigation: This is the process used for transitioning between different router states. To trigger a navigation, you must check the following actions;
- Click on anchor tags, represented with (<a>), to initiate user interaction with router link directives.
- Use the router.navigate method for pragmatic navigation for sending users to specific routes.
Angular router helps with Angular advanced navigation control by three methods;
-
- Matching the existing URL with the defined router state.
- Destroying components connected with the previous state.
- Create and display components connected with the newly elected state.
- Lazy Loading: It’s a technique we use in Angular development when the task is to load JavaScript components when a particular route is activated. As a result, the end-user will experience a better application load time and speed as the application is split into bundles.
I would say that it’s an optimization technique. What happens in the default mode, means without lazy loading, Angular will load all application modules, including the components associated with them. However, with lazy loading, it’s possible to suspend loading certain modules and their components until and unless they are actually needed by the user.Do the following to achieve this functionality;- Define feature modules that include a specific set of functionalities and components.
- Use the loadchildren property in the router configuration that will specify a function. This will load the feature module and its route dynamically.
// lazy-loading.module.ts import { NgModule } from ‘@angular/core’;
import { CommonModule } from ‘@angular/common’;
import { LazyComponent } from ‘./lazy.component’;
@NgModule({
declarations: [LazyComponent],
imports: [CommonModule],
exports: [LazyComponent]
})
export class LazyLoadingModule { }
The above code script is for a simple module, LazyLoadingModule, with LazyComponent representing how the feature module will be lazy-loaded.
In essence, lazy loading reduces the initial app bundle size, leading to an improved initial load time and performance. Plus, it improves Angular’s lazy loading code while separating functionalities into different components.
The three pillars of routing in Angular, Router States, Navigation, and Lazy Loading, help create a robust and efficient routing system.
Angular Forms | Creation, Validation, and Submission
Forms, when used in Angular, will let you provide a structured way to handle user input, and it works exceptionally well with web applications. Angular form creation also has built-in validation, data binding, and error handling.
Developers use two types of forms when working with Angular;
- Angular Template-Driven Forms: A basic type of form suitable for developing a limited number of fields and working with a simpler validation. Every field in this form is represented with a component class. You can import FormsModule from the @angular/forms package.
Key concepts related to Template-Driven Forms:- ngForm Directive: Representing an Angular form, this exposes methods and properties related to the form to validate and manipulate data properties.
- ngModel Directive: It’s used for two-way data binding between different forms of control elements.
Template-Driven Forms Validation: According to Angular validate form input, you will get different validator properties applicable to form controls based on indicating their validation state.
- Touched: A boolean number is seen if the control is touched.
- Untouched: The opposite result of touched; no boolean value is seen.
- Valid: Another boolean value verifying whether the control value is valid.
- Invalid: It’s the opposite of the valid response.
After this, here’s another important bit of information related to Angular form validation techniques and validation Directives. These directives can be used with ngModel to perform validation, including;
- Required: A directive to ensure the control has a non-empty value.
- Min and Max Length: Used to ascertain the minimum and maximum length of a value.
- Pattern: Used to validate a value for a regular expression.
- Email: It ascertains shown value is a valid email address.
- Reactive Forms: Angular has two types of forms, Template-driven and Reactive. Template driven forms are based on directions, but reactive forms, on the other hand, are model-driven.Reactive forms offer better flexibility and control for handling inputs, especially those whose value changes over time. A unique property you will observe while working with Reactive Forms is how it manages to maintain the integrity of the model during changes. This happens because each change in the form state returns a new state.
<!– Template-driven form –> <form #form=”ngForm” (ngSubmit)=”onSubmit(form.value)”>
<input type=”text” name=”name” ngModel>
<button type=”submit”>Submit</button>
</form>
<!– Reactive form –>
<form [formGroup]=”form” (ngSubmit)=”onSubmit()”>
<input type=”text” formControlName=”name”>
<button type=”submit”>Submit</button>
</form>
Reactive Forms in Angular use observables and data streams meant to manage the validations state. This is done with some built-in Angular form validation techniques (which we have discussed above) and custom validators.
Developers tailor validators to implement a specific validation logic, offering more granular control over the process. A custom validator takes a FormControl argument and sends back a validation error object in the form of a key string or a null value, depending on the validity of the control.
These customized validators are used to validate values depending on the multiple forms of control. Plus, they can also perform asynchronous validation using external services.
To complete the validator functions, developers use built-in validators and custom logic. Using the Validators.compose function, you can accept multiple validators and fuse them for adding multiple validation checks.
Multiple Environment Setup in Angular
Dubbed configuration environments, a multiple environment allows Angular developers to customize the app’s behavior according to the deployment context. With this, you can build Angular apps for development, staging, and production. As a result, you can have separate configurations for different app environments and ensure that they behave in each environment as intended.
By default, Angular CLI can create two environment files, environment.ts and environment.prod.ts. But you can also create custom environments by adding new files in the directory. For instance, for staging environments, use the command environment.staging.ts.
Every environment file exports an ‘environment’ object. This object has configuration variables specific to the environment, including API URLs, feature flags, among other aspects.
Some benefits of a multiple environment include better app maintainability, enhanced security, and flexible testing, along with feature flag management. Here’s how to set up multiple environments in Angular;
- Environment Files: Type in the Angular CLI command, ng generate environments environment to build environments folds in the root directory. From here, you can generate two default files, environment.t,s and environment.prod.ts, for the base and production environment configuration.
- Define Environment Variables: In each of the environments, you can create variables to hold configuration values. However, note that these values will be specific to the environment.
// environment.ts export const environment = {
production: false,
apiUrl: ‘http://localhost:3000/api’,
logging: ‘debug’,
featureFlagA: true
};
// environment.prod.ts (production config) export const environment = {
production: true,
apiUrl: ‘https://your-api-endpoint.com/api’,
logging: ‘error’,
featureFlagA: false
};
- Injecting Environment: Moving on, you have to inject environment files into Angular components and services at points where you will need to use configurations. You have to inject the environment using the @Inject decorator and environment token.
import { Component, Inject } from ‘@angular/core’; import { environment } from ‘./environments/environment’;
@Component({
selector: ‘app-my-component’,
template: `
API endpoint: {{ environment.apiUrl }}
`
})
export class MyComponent {
constructor(@Inject(‘environment’) private env: any) {}
ngOnInit() {
console.log(this.env.logging); // Access environment variables
}
}
- Configuring the App Build Process: To start the build process, you must modify the angular.json file to specify an environment file to use for build commands. The code snippet example is for the Production and Development environment.
“architect”: { “build”: {
“options”: {
“configuration”: “production” // For production build
}
},
“serve”: {
“options”: {
“configuration”: “development” // For development server
}
}
}
Following this system, you can build a solid multiple environment management system in Angular application. However, to build a smooth system, ensure you are adding proper configuration in different deployment stages.
Observables and RxJS in Angular
Observables in Angular are used to handle asynchronous operations and data streams. A part of the Reactive Extensions for JavaScript (RxJS) library, these are used for several common operations. Moreover, they provide a way to subscribe to and receive notifications in the application when new data or events take place. This allows developers to make changes to the application in real-time.
But what’s the purpose of using RxJS observables with Angular? Here’s a short version. By default, JavaScript is a single-threaded language, which means the code is executed line-by-line. As a result, when one HTTP request is sent, it must be completed before the next one can be executed.
Following this process, you cannot create fast-performing applications wherein it’s natural to have different requests and operations executed at once. This is where asynchronous coding comes in with its non-blocking execution of the code. But to make these asynchronous HTTP requests, you need to implement observables in Angular.
While we are on this topic, I would like to focus on observables handling data streams, which represent the sequences of values emitted over time in Angular. The observables handle this in six steps. But before we go to the steps, let me cover a few key concepts you will need to understand the steps.
Concept | Description |
Observable | It’s an object representing a stream of values or events emitted over time. |
Observer | This mentions an object subscribing to an observable for receiving emitted values. |
Subscription | It’s the connection or bridge between the observable and the observer, allowing the latter to receive notifications and unsubscribe once the task is complete. |
Operators | These represent the functions for transforming or manipulating observable data streams in different ways. |
Here’s how it works;
- Creation: Create observables with functions like of() and from(). You can also use operators like interval() or timer() to create them. Their purpose is to describe the how and when the valued will be emitted.
- Subscription: Once created, an observer will subscribe to the observable with subscribe(), creating a connection between them and enabling the observer to receive emitted values.
- Emission: Based on the defined logic, the observable emits values in the form of data or events.
- Notification: With each event, the observer is notified with the details of the emitted value with the next() callback function. And this happens within the subscription.
- Completion: Although this step is optional, I recommend you perform this as well. Completion is when the observable completes emitting values followed by optionally notifying the observer through a complete() callback.
- Error Handling: Another optional function, where after coming across an error, the observer received a notification through the error() callback function.
- Unsubcription: With the function complete and observer no longer capable of receiving values, it can unsubscribe with the unsubscribe() method. This is important to prevent memory leaks.
Asynchronous data handling is one of the clear benefits of using observables. But there are others as well, like;
- Composability: As you implement observables in Angular, they can be combined using operators to complete transformations or combine multiple data streams; it can help you write reusable and maintainable code.
- Reactive Programming: Following a reactive approach provided by observables, it’s easier to define how you wish to react to emitted values.
Most Common Use Cases for RxJS Operators in Observables
With RxJS, which is a JavaScript-compatible library, you can provide a suite of operators catering to different scenarios.
Operator | Description |
Map | Use this function to transform every emitted value from the observable source. |
Filter | After adding a Filter, you can control emitted values as they have to pass a test by a provided predicate function. |
switchMap | switchMap emits values only from the most recently projected Observable after projecting each source value to an Observable. |
catchError | This operator is used to manage errors emitted from the observable source. |
combineLatest | As the name suggests, it combines multiple observables and emits the latest values from each. |
The following code snippet is for using RxJS to handle HTTP requests with an observable;
import { HttpClient } from ‘@angular/common/http’;
import { Observable } from ‘rxjs’; import { map } from ‘rxjs/operators’; // Import the ‘map’ operator interface User { name: string; email: string; } @Component({ selector: ‘app-user-details’, template: ` <h1>User Details</h1> <p *ngIf=”user”>Name: {{ user.name }}</p> <p *ngIf=”user”>Email: {{ user.email }}</p> ` }) export class UserDetailsComponent { user: User | null = null; constructor(private http: HttpClient) {} ngOnInit() { const userId = 1; // Replace with your user ID this.getUser(userId) .pipe( // Use the pipe method to chain operators map(user => (this.user = user)) // Transform the emitted user data ) .subscribe(); // Subscribe to the observable } getUser(userId: number): Observable<User> { return this.http.get<User>(`https://api.example.com/users/${userId}`); } } |
Top Angular Features You Should Checkout Before Development
Hailed as a powerful and flexible framework for web development, Angular holds its position resting on the shoulders of its amazing features. These features will make your development experience simpler, easier, more efficient, and, of course, faster. Let’s go through some of these below;
-
HTTP Client
Angular’s HttpClient simplifies HTTP request handling and responses. This happens through four key elements;
-
- Request methods
- Interceptors
- Error handling
- Observables
The request methods used here return observables, leading to asynchronous response management. Plus, it uses RxJS observables (more details on this will come later) again to handle asynchronous HTTP operations.
-
Hydration
Hydration is a technique used in Angular development to enhance SPA’s performance. This happens because Hydration utilizes both server-side and client-side rendering.
The requests sent to the Angular application page are initially rendered server-side. Once the pre-rendered request is sent to HTML, client-side rendering takes over.
With both the rendering sides working simultaneously, Hydration leads to an improved first contentful paint (FCP). Websites built with this practice are easier
-
Deferrable Views
Deferrable View was launched in Angular 17+ and is already making headlines for optimizing the initial load time of the web application. Using this feature, developers can declaratively delay loading sequences of specific sections of the template provided during development.
As a result, it reduces the initial bundle size that needs to be downloaded by the users during engagement. Moreover, it offers improved perceived performance in a way where the core content of the page is viewed immediately, but the non-critical sections load asynchronously in the background.
-
Image Optimization
Introduced in Angular 15, developers can use the NgOptimizedImage directive to optimize image loading. As a result, it’s now easier to optimize application performance as well, and this directive comes with several benefits;
-
- Intelligent lazy loading
- Optimized CDN configuration
- Built-in validation and warnings
- Fill mode for flexibility in sizing
- Better support for custom loaders
Using the NgOptimizedImage directive, you get a powerful suite of features leading to optimized image loading and performance.
-
Testing
As testing is crucial for building robust applications in Angular, you can use it to make changes to the application, ensuring that it behaves as intended. In Angular, you can conduct the following;
-
- Unit Testing
- End-to-End Testing
- Integration Testing
Effective testing helps you build better code for the application while making sure it is maintainable, secure, scalable, and readable. Moreover, well-tested code instills better confidence in the developers, helping them experiment and build better features.
-
Internationalization
Angular has built-in support for internationalization (i18n) and is represented in the @angular/localize package. A simple benefit of this feature is that you can translate your application into multiple languages with this feature.
To implement this feature, follow these steps;
-
- Install the @angular/localize package and define the languages you want the app to support in angular.json in the i18n section.
- Again, use the i18n attribute available on HTML elements to mark the text you need translated. If required, provide additional context with the meaning|description format.
- Extract the message for translation with the ng extract-i18n command, and this will convert the text into XLF files. These files are compatible with the translators and are sent for localization.
- As the extracted files are translated into the target language, you can put them in the src/locale directory.
-
Animation
In Angular, you get a suite of robust features to add animations to web applications. Follow these steps to add setup and animations;
- Start by important the BrowserAnimationsModule to the root module and this will allow you to add animations to the application.
- Declare the animations in the @component metadata of the component related to the feature or area where you want to add animations.
- Angular provides a few functions to define animations, including
-
-
- trigger()
-
-
-
- state()
-
-
-
- animate()
-
-
-
- transition()
-
-
- With @triggerName syntax, you can add a point to the animation trigger in the component template.
- Using event bindings like @triggerName.Start and @triggerName.Done you can listen for animation events, meaning you can hook into the animation lifecycle and provide additional logic.
-
Service workers
Service workers are additional scripts that run in the background of the user’s browser. These scripts are intermediaries between the web application built with Angular and the network, and they have several benefits.
- Caching Static Objects: With the caching capabilities of static assets and API responses, service workers can provide cached content even when the user is offline.
- Push Notifications: These workers enable applications to receive push notifications from different servers. This means they can help keep the users informed, even when the application is not running.
- Background Synchronization: Service workers have proven to be an effective tool in synchronizing data between applications and servers. This ensure that changes made when offline synchronize after internet connectivity regains.
-
Web worker
Another powerful feature of Angular is that web workers are used to run computation-intensive tasks in the background separately from the main application thread. In essence, they offload tasks with heavy processing requirements to maintain a smooth user experience. As a result, the main thread isn’t blocked, which otherwise can freeze due to unresponsive UI.
Web workers are mostly used in Angular for image processing, data analysis, and long-running tasks that can eat up a lot of computation power. To generate a web worker in Angular, you can use ng generate web-worker <name>. In this, replace <name> with the desired worker name.
-
Server-Side Rendering (SSR)
SSR allows developers to pre-render web pages on the server before they are sent to the client, which brings several benefits;
- Faster Initial Load Times: Pre-rendering the initial page on the server reduces the time it takes to load the first page. Doing so leads to a smoother user experience.
- Better SEO: Search engine crawlers will find it easier to crawl websites enabled with SSR, which increases their probability of ranking among the top SERPs.
- Angular Universal: A dedicated module with SSR functionality, its handles the SSR process and hydration of the same with the application’s client side.
-
Prerendering
Also called Static Site Generation (SSG), it’s a process where you can render web pages to static HTML files and is practiced during the build process. There are two types of prerendering, including Static and Dynamic.
Static prerendering is when all routes are prerendered during the build process, after which static HTML files are served to the users. Second is Dynamic prerendering, which prerenders specific routes based on a configuration file or script.
Prerendering comes with a couple of benefits;
- Better Performance: Prerendering reduces Time to First Byte (TTFB) as it serves HTML files, which enhances the user’s overall experience.
- Reducing Server Load: Since static HTML files require less server processing than dynamic pages, it lowers the load on the server leading to faster performance.
-
Angular Libraries
Libraries are reusable packages of code that encapsulate a functionality, component, service, pipe, or any other building block you need for Angular applications. Using these libraries, you will benefit from code reusability and improved maintainability.
Plus, you can get feature encapsulation, which prevents unintended side effects or conflicts. Independent versioning, grants a dedicated versioning cycle to the libraries. This enables updates without affecting the primary application service.
Why Choose Mobmaxime for Angular Development?
Angular is a phenomenal framework we use to build applications that continue to impress and amaze. As the top Angular development company, our expertise in working with Angular and the customized approach we follow for every project enables us to build solutions that will defy all odds and help your business breach every barrier and achieve its goals, including yours.
Whether you need consultation on why to choose Angular for your next project or need to hire the best Angular development company, go for Mobmaxime. Leveraging our years of experience and expertise in using the framework, we conduct an in-depth analysis before of the requirements before creating the development strategy and work on the project.
So, if you are looking to hire a dedicated Angular developer, you know who to contact.
Join 10,000 subscribers!
Join Our subscriber’s list and trends, especially on mobile apps development.I hereby agree to receive newsletters from Mobmaxime and acknowledge company's Privacy Policy.