Dependency Injection in Angular: Best Practices for Organizing Services
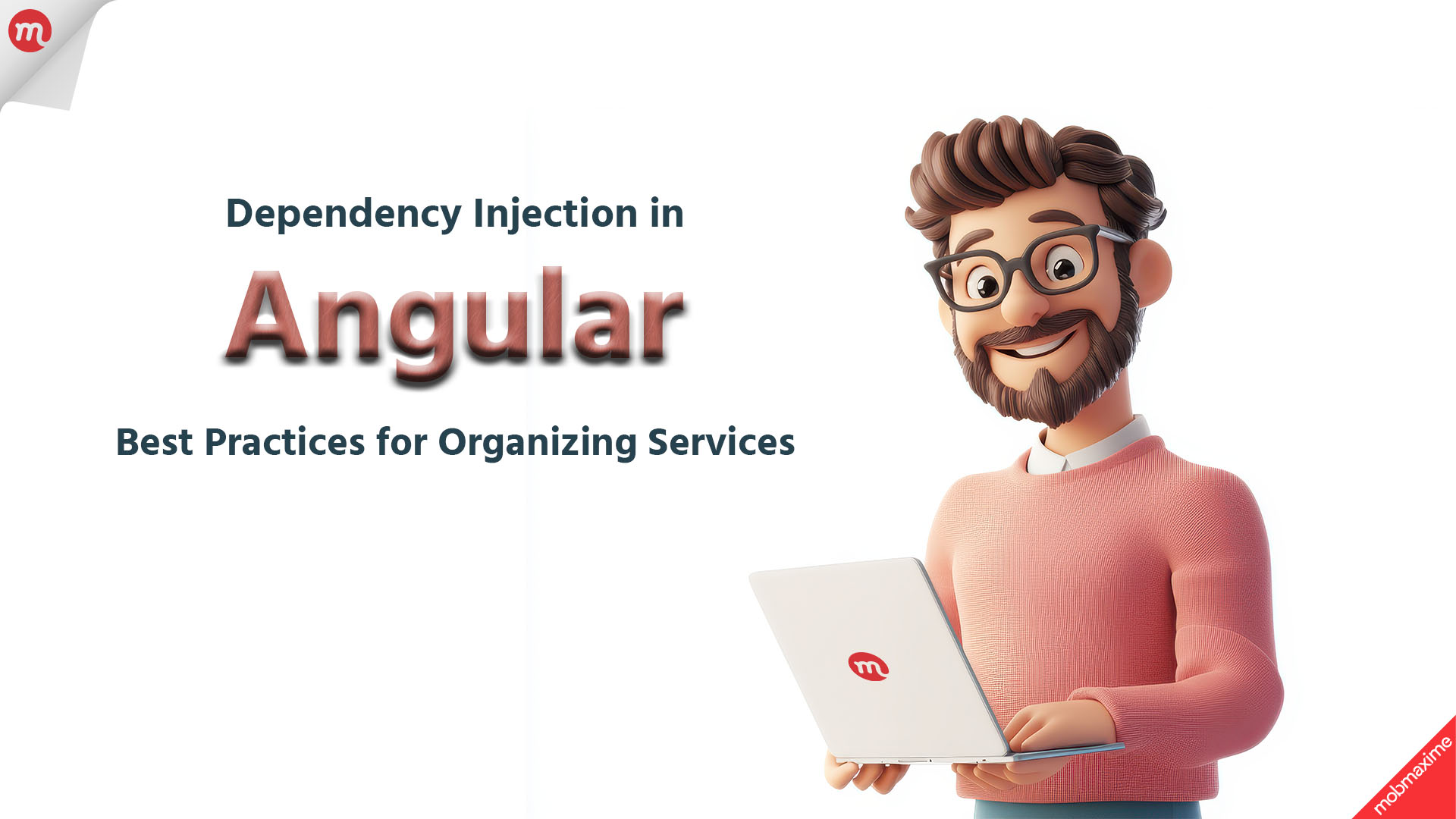
Dependency Injection (DI) is a core design pattern in Angular that enhances modularity and simplifies service management. When injected into components, dependencies, such as services, reduce tight coupling and improve code organization.
As a result, developers can manage shared services efficiently across different parts of an application while creating cleaner, more maintainable code. Dependency injection is also crucial for testing, which helps improve the overall testability of the application.
To understand how dependency injection fits into the broader Angular ecosystem, explore our detailed guide on Angular development.
What are Services in Angular?
Services in Angular are meant to organize and manage reusable logic throughout an application. It’s a class developer that integrates into the code to perform a specific task, such as retrieving data from an API, managing user sessions, or encapsulating business logic that can be shared across multiple components.
Angular services facilitate clean separation of concerns to ensure components stay focused on UI logic, particularly when sharing non-UI tasks with services. Using this approach, a top Angular development company can build a more modular, maintainable codebase, which is especially important for large applications.
Use Cases for Angular Services
Angular services are used in a variety of scenarios to enhance code reuse and maintainability:
- Data Fetching: Services manage HTTP requests to extract or transfer data from/to a server using the HttpClient function.
- State Management: Services store and manage the app’s state to make sure it remains consistent across components.
- User Authentication: The services we use in Angular development manage user authentication and authorization, handling login sessions, tokens, etc.
- Business Logic: Services can encapsulate business logic, without which it can easily clutter up components, improving readability.
Integrating these tasks into services, developers can write DRY (Don’t Repeat Yourself) code, allowing multiple components to access the same logic without redundancy.
What Types of Services are Used in Angular?
Dependency injection in Angular offers flexibility in how services are provided and managed during the development phase. This influences how services are scoped, instantiated, and reused throughout an application.
Type of Services | Description |
Singleton Services | Singleton services are executed only once and are also shared with the entire application. Considered as the most common type, they provide a root injector by default with providedIn: ‘root’ in the service decorator.
Doing so means that this service is shared by all components and modules. Singleton services are ideal for managing global state or shared logic that doesn’t need to be duplicated |
Multiple Instances | Multiple instance services are executed in the development process at the component or module level. This means a new instance of the service is created for each element that requires it.
This is useful when developers need service isolation or separate instances with different states for different components. By providing a service in a specific module or component, it’s easier to limit the scope and prevent it from being shared across the entire application. |
You can carefully control how and where services are instantiated using services and their instances. Singleton services are ideal for scenarios where shared logic is needed globally, while multiple instances of services can be used when components require individual service states.
But How Do Services and Dependency Injection in Angular Relate?
Angular’s DI system is fundamental in deciding how services are managed and provided throughout the application. These services register with Angular’s injector, which creates instances of the service and delivers them to the required components.
Using the @Injectable() decorator, the service informs Angular that services can be injected into components or other services. By using DI, Angular manages the lifecycle and instantiation of the required services while ensuring developers won’t have to manually handle dependencies.
Using Angular dependency injection, you can ensure the appropriate version of the service is injected based on its scope, hence creating a powerful tool for maintaining service instances across the application.
Both services and dependency injection work together to promote modularity and reliability in application development while helping build a clear and modern architecture.
How Dependency Injection in Angular Works?
Dependency Injection (DI) is a powerful mechanism enabling classes to receive dependencies from external sources instead of creating them internally. To effectuate these systems, Angular developers must understand providers and injectors and how they can help manage the lifecycle of services and deliver them to components and other services.
What are Providers and Injectors?
Providers instruct Angular on creating an instance of a service. At the time of defining a service class in Angular development, register it with a provider. This helps ensure that Angular’s dependency injection system can know how to supply it when required.
The injector helps create and maintain the service instances based on the provider configuration. It helps deliver instances of the requested service as required.
When working with Angular, you must understand a hierarchical injector system. This is when services can be analyzed at different levels (root or component-level), leading to effective management.
In addition to these, with Angular’s DI you can inject services at root or component levels.
- Root-level injection facilitates a global instance through providedIn: ‘root,’ which is shared by all components.
- Component-level injection provides separate instances for each component, offering isolation.
When choosing among the two, go for root-level for global resources and component-level for local state.
Understanding the @Injectable() Decorator
The @Injectable() decorator is essential for Angular’s DI system, and it’s used to mark a class as a service so that it can be injected into other components or services.
Without using the Injector function, Angular cannot process how to instantiate the service and inject it into the required segments of the application you are developing.
@Injectable({
providedIn: ‘root’
})
export class UserService {
constructor() { }
}
Another reason for using the @Injectable() decorator is to instruct Angular which services (dependencies) the class required. For instance, if one service depends on another service, Angular DI is used to execute that dependency automatically.
Best Practices for Using Services in Angular Dependency Injection
One of the areas where you will need to follow Angular dependency injection best practices is in the implementation of services. Before we move to the best practices, understand that it’s crucial to define clear service boundaries to ensure that each service-specific and laser-focused responsibility.
What are Service Boundaries?
In development, service boundaries put up limits or constraints defining the scope and responsibilities of a specific service. These services are;
- Cohesive, meaning they focus on a specific set of functionalities.
- They are loosely coupled, ensuring they have minimal dependencies on other services.
- Services are also scalable, which ensures they can meet the increasing demand without affecting other services.
This practice adheres to the Single Responsibility Principle (SRP), which states that a service should have only one reason to change. By keeping services focused on a single task, your code becomes more modular, maintainable, and easier to test.
-
Use Module Providers for Large Applications
Organizing services by feature modules is one of the best approaches to follow for larger applications. In this, you will basically provide all services globally and break them down into module-specific providers.
Once implemented, this approach will improve the app’s scalability and reduce the app’s memory footprint. Not only this, using services within feature modules, the Angular app will ensure that the service is only instantiated when the related module is loaded. As a result, services won’t be unnecessarily created and executed when they are not needed, effectively reducing memory consumption.
Another benefit of using module providers is limiting service scope to specific modules to prevent memory leaks. The rationale behind this is that if a service is not used across the entire app, why provide it at the root level, where it stays active throughout the application’s lifecycle.
When using module-level providers, developers will allow Angular to manage the service lifecycle, ensuring they are disposed of when the module is unusable.
-
Compare Root Injector and Component-Level Injector
In Angular, you have two options to register services at primary levels: Root Injector and Component-Level Injector.
- Root Injector (Global): The services provided at the root injector level are singleton services, and they are shared across the entire application. It’s beneficial for services that are supposed to manage global state or resources, like;
- Authentication;
- Configuration;
- API services.
Given this, you must also use global services judiciously to avoid performance issues. Issues may arise with unnecessary service instances being created and shared in the entire app.
- Component-Level Injector (Local): When providing services at the component or module level, you may have to generate a separate instance of that service. This is especially relevant when you need to provide for each instance of the component or module.
This is ideal for development scenarios where different parts of the application need isolated instances of the service. Suppose the application you are building has every component managing its own state independently. By doing so, you can ensure to facilitate a new instance of the service for each element to prevent conflicts.
Proper scoping of services ensures that unnecessary service instances are not created, leading to more efficient memory usage and application performance.
-
Using Lazy Loading with Dependency Injection
As you already know, lazy loading is one of the key optimization strategies in Angular, especially for large applications. With this approach, you can load modules and services only when they are required, which is helpful in reducing the initial load time of the application.
By combining Lazy Loading with Angular dependency injection, services can be instantiated only when the corresponding module is loaded, hence, optimizing resource usage. Doing so delays the starting of heavy services that’ll fetch large datasets or handle complex logic that are not loaded at the start, albeit they load when the user navigates to the relevant part of the application.
For implementing lazy loading and optimizing dependency injection Angular uses loadChildren property from the routing configuration. This property will instruct Angular to load the requested module only when the route is accessed.
As a result, you can ensure that the application does not work under stress and remains lightweight, even when the app size grows.
Advanced Dependency Injection Techniques Angular Developers Must Know
Using advanced concepts in dependency injection is meant to enhance the application’s effectiveness and flexibility. Using these advanced techniques improves the application’s modularity, and reusability and enhances the scope for its testability.
-
Use Multi-Provider Tokens in Angular
Multi-provider tokens in Angular will allow you to register multiple services under one token. This grants flexibility in the way you inject and use services. Such a function is particularly useful when you must inject various implementations of a service and also use them interchangeably.
For example, if you have multiple loggers (e.g., ConsoleLogger and FileLogger), you can provide both of them under the same multi-provider token and then inject them as an array in your component or service.
Code Script for when you have to configure multi-provider tokens: import { InjectionToken } from ‘@angular/core’;
export const LOGGER_SERVICE = new InjectionToken(‘LoggerService’);
@Injectable()
export class ConsoleLoggerService { /*…*/ }
@Injectable()
export class FileLoggerService { /*…*/ }
// Provide both services under the same token
@NgModule({
providers: [
{ provide: LOGGER_SERVICE, useClass: ConsoleLoggerService, multi: true },
{ provide: LOGGER_SERVICE, useClass: FileLoggerService, multi: true },
],
})
export class AppModule {}
Once this is done, use the following code to inject loggers into the component
@Component({ selector: ‘app-root,’
template: ‘<p>Check the console for loggers.</p>’
})
export class AppComponent {
constructor(@Inject(LOGGER_SERVICE) private loggers: Array<any>) {
this.loggers.forEach(logger => logger.log(‘Logging something’));
}
}
-
Using Injection Tokens for Non-Class Dependencies
At times, you may have to inject non-class dependencies like configuration settings, strings, or objects into the Angular application services or components. While Angular won’t allow you to inject primitive types like strings or numbers, there’s a way around this limitation, which is through InjectionToken.
An InjectionToken is a generic and strongly typed token you can use to represent non-class dependencies. For example, you might have a configuration object with environment-specific settings that you want to inject; use this code script to create InjectionToken and use it for adding configuration data.
import { InjectionToken } from ‘@angular/core’; export const APP_CONFIG = new InjectionToken(‘app.config’);
// Example configuration object
export const AppConfig = {
apiUrl: ‘https://api.example.com’,
timeout: 3000
};
// Provide the configuration object
@NgModule({
providers: [{ provide: APP_CONFIG, useValue: AppConfig }],
})
export class AppModule {}
Once done, use the following code to inject the configuration into a service or component;
@Injectable() export class ApiService {
constructor(@Inject(APP_CONFIG) private config: any) {
console.log(this.config.apiUrl); // Accessing injected configuration
}
}
-
Use Self-Decorators and Optional in Angular Development
In the Angular dependency injection system, you can use several useful decorators, such as @Optional() and @Self(), to have better control over how services are injected.
- @Optional(): The @Optional() decorator allows you to inject a dependency that might not always be available. If the service isn’t provided, Angular will inject null instead of throwing an error. This is useful for optional dependencies or fallback scenarios.
@Injectable() export class ExampleService {
constructor(@Optional() private logger: LoggerService) {
if (this.logger) {
this.logger.log(‘Logger is available.’);
} else {
console.log(‘No logger provided.’);
}
}
}
- @Self(): The second decorator you can use is @Self() which tells Angular to look for the dependency in the current injector at the component level. If the dependency is not found at the specified level, the application will show an error. This is useful when you want to ensure that the dependency is provided at a local level and not inherited from parent injectors.
@Injectable() export class ExampleService {
constructor(@Self() private localService: LocalService) {
// Ensures that LocalService is injected from the component’s local injector
}
}
Both decorators offer control over how and where dependencies are injected, giving you flexibility in managing service instances in more complex scenarios.
- @Optional(): The @Optional() decorator allows you to inject a dependency that might not always be available. If the service isn’t provided, Angular will inject null instead of throwing an error. This is useful for optional dependencies or fallback scenarios.
Conclusion
A well-organized services structure is the backbone of a scalable and maintainable Angular application. Knowing Angular dependency injection best practices such as you can create modular, efficient, and high-performing apps.
Moreover, using advanced concepts ensures that your application always performs optimally. For a broader understanding of Angular development, including essential concepts like components, directives, and forms, we recommend checking out our comprehensive guide on Angular development.
At Mobmaxime, we deliver cutting-edge, scalable, and customized Angular solutions to help you meet your business needs. Our team of expert developers can help you implement best practices in dependency injection and service organization to enhance the performance and maintainability of your applications.
Join 10,000 subscribers!
Join Our subscriber’s list and trends, especially on mobile apps development.I hereby agree to receive newsletters from Mobmaxime and acknowledge company's Privacy Policy.