Exploring Angular Modules: A Comprehensive Overview
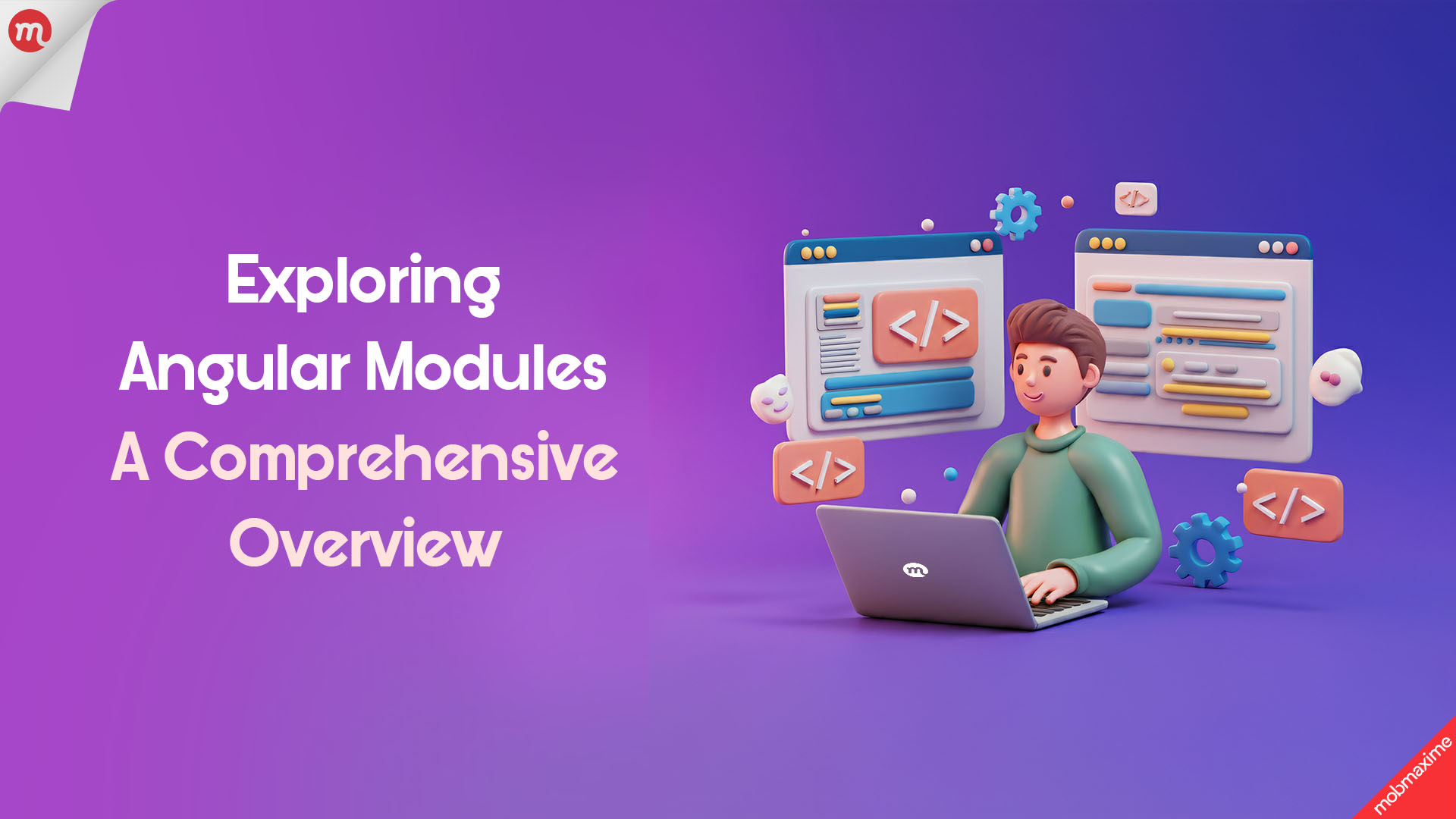
Angular modules help organize an application into cohesive blocks of functionality. Each module has a particular purpose, which can be bundling together components, directives, pipes, and services connected to your building functionality.
Every Angular application has at least one module, which is the root module. However, more modules are added as the application grows, and these additional modules help maintain order.
This means that modules are crucial for building large-size and scalable applications. Since they help break down large, monolithic code scripts into manageable, reusable pieces. It improves maintainability, leading to better team collaboration.
At Mobmaxime, we keep our projects clean by logically separating functionality with the help of modules. For instance, with feature modules for specific sections like authentication or user management, we ensure the codebase is easier to navigate and extend as the project evolves.
Let’s learn more about the Angular module’s best practices and their importance.
Understanding Modules in Angular Application Structure
Defined by the @NgModule decorator, the Angular module is a class function we use to organize related components, services, directives, and pipes into an interconnected block of functionality. Angular NgModule framework works as a container for the application elements, ensuring they work according to the predetermined context.
In other words, we can say it’s a deployment sub-set you can use to write your whole application and split it into smaller parts for better management.
Key components we use in Angular NgModule Framework
- Declarations: Declarations are used to list components, directives, and pipes that belong to this module. They are essentially building blocks of the application and help define its structure and behavior over the user interface.
- Imports: This function allows importing the needed functionality. It’s often used for reusing the code you extractable from other parts of the application and helps ensure the codebase is maintainable.
- Exports: Using Exports, we provide components, directives, or pipes that should be made accessible to other modules. Doing so will make the exported components available for functionality to different parts of the application.
- Providers: Providers are used to define services that are to be made available throughout the module or the entire application. These are services that are responsible for handling business logic and data management.
- Bootstrap: This function ensures the main application component gets bootstrapped when the module is loaded. This component represents the entry point of an Angular application while defining its root component.
For an easier understanding, let me take the help of an analogy. Consider modules as rooms in a house, where each room serves a different purpose, including a kitchen for cooking, a bedroom for resting, and so on.
Similarly, Angular modules unite related code to serve a specific functionality, while keeping everything organized and easy to manage.
Reasons to Use Modules for Angular Application Structure
As a top Angular development company, we are always looking to find new ways to use modules to improve development outcomes. Here are some reasons to use modules;
- Modularization: The modules we use help divide a large codebase into smaller, more manageable units, effectively making large application development easier.
- Organization: Since Angular modules help unite related components, services, and pipes, it improves the developer’s understanding while allowing them to improve code readability and maintainability.
- Scalability: As a result of modularization and organization, modules facilitate application growth while helping create a modular architecture.
- Reusability: Modules can be shared across different projects or even within the same application, reducing code duplication and improving development efficiency.
- Dependency Management: Modules define dependencies between different application parts, ensuring that components, services, and pipes have access to the necessary resources.
- Testing: Since modules can be tested independently, we can simplify the testing process and improve code quality.
- Code Separation: Modules are used in Angular to separate concerns; again, this makes the codebase easier to understand and maintain.
- Lazy Loading: Angular allows for the lazy loading of modules, which can improve application performance by delaying the loading of modules until they are needed.
- Feature-Based Development: With Angular modules aligned with specific features or functionalities, it helps build a better, high-speed application that is also easy to maintain, in terms of development and maintainability.
- Collaboration: Modules can be developed and maintained by different teams or individuals, promoting collaboration and improving development efficiency.
Based on years of experience, modularizing application code is always preferable for the development, design, testing, and deployment teams. It leads to faster builds and improved maintainability.
For example, by lazy-loading specific feature modules, I’ve seen significant performance improvements, especially in large apps. This approach also ensures that as the application grows, the code remains clean, and adding new features doesn’t require touching every part of the app, just the relevant module.
Deciphering Core Modules and Feature Modules in Angular
To fully follow the Angular module’s best practices, you need to have a good understanding of Angular’s code and feature modules. Here are the important bits you need to know about them.
-
Core Module
The Core module defines services, singleton objects, and components. However, these are the elements that will only be instantiated once and used across the entire app.
For instance, services with application-wide functionality like authentication, logging, or routing should be added in code modules. Moreover, a core module is typically imported only in the root AppModule and never in any other module to avoid multiple instances of the same service.
@NgModule({ providers: [AuthService, LoggingService],
imports: [CommonModule]
})
export class CoreModule { }
-
Feature Modules
Feature Modules are useful in bringing together related components, services, and other functionalities to facilitate operations in a specific application part.
As an essential part of the Angular application structure, feature modules are useful when you want to integrate user management or product management into the app.
Each Feature module takes care of distinct features of the application, and it can also be imported into the root module or other feature modules as and when required.
@NgModule({ declarations: [UserComponent, UserProfileComponent],
imports: [CommonModule],
})
export class UserModule { }
-
Shared Modules and Lazy-Loaded Modules
Shared modules in Angular are used to store common functionality that you want to reuse with different Feature Modules. These usually include shared components, directives, and pipes, which you can import into multiple modules and ensure nothing is duplicated.
@NgModule({ declarations: [HeaderComponent, FooterComponent],
imports: [CommonModule],
exports: [HeaderComponent, FooterComponent]
})
export class SharedModule { }
Lazy-loaded Modules are feature modules that you can load on-demand. This means they won’t load when the application starts, which improves the app’s performance and speed. This improves performance by reducing the initial bundle size.
const routes: Routes = [ { path: ‘user’, loadChildren: () => import(‘./user/user.module’).then(m => m.UserModule) }
];
Usage of Core vs Feature Module
You should use a Core module for services and components that need to be shared across the entire app (like authentication or error handling). On the other hand, feature modules are best used when organizing code related to specific features, such as user profiles or product listings. This division keeps your app scalable and maintainable as new features are added.
Step-by-Step Process to Create Module with Angular CLI
When creating Angular modules, start by grouping related components, services, and other resources into modules. By doing so, you will maintain a clean and scalable codebase.
With Angular’s CLI, setting up a module requires some key steps, but it also has some common pitfalls you need to avoid.
- Open your terminal: Navigate to the root directory of your Angular project.
- Run the CLI command: Use Angular CLI to create a module through the following command: ng generate module my-module
Using this command, you will create a folder named my-module containing a my-module.module.ts file. - Declare components: You can declare components, directives, and pipes specific to this module, ng generate component my-module/my-component. When using this command, ensure that the new component is automatically added to the declarations array in my-module.module.ts.
- Import necessary modules: Now, within the @NgModule decorator, you must add the necessary imports.
@NgModule({ declarations: [MyComponent],
imports: [CommonModule],
exports: [MyComponent]
})
export class MyModule {}
- Utilize the application module: Once done, you can now use the module in your application and import it to the root AppModule or any relevant feature module using the following code script.
import { MyModule } from ‘./my-module/my-module.module’; @NgModule({
imports: [MyModule]
})
export class AppModule {}
Common Mistakes to Avoid while Building Angular Modules in CLI
Working with Angular may be easier than with other similar platforms, still mistakes can still happen. Leading the top Angular development company, even we have to deal with challenges at some points. But what good will our experience bring if we cannot share how to achieve the best results with Angular CLI? So here are the common mistakes to avoid when using Angular.
- Duplicating imports: Never import similar modules, in the root and a feature module as it can lead to issues. Duplicating imports can lead to;
- Creating multiple instances of Singleton services;
- Unnecessary memory usage and leaks;
- Unexpected behavior can erupt when working with dependency injection;
- Network can behave erratically due to configuration duplication.
So, avoid importing CoreModule in feature modules to prevent creating multiple instances of singleton services.
- Forgetting to export components: If you want to use a component or directive from a module in other modules, don’t forget to add it to the export array.
- Overloading the root module: Keep your root AppModule lightweight. Delegate feature-specific components and services to feature modules instead of overloading the root module.
Angular Modules Best Practices You Must Follow
When it comes to properly organizing Angular modules, developers must ensure that they maintain a clean and scalable application, especially when it grows. Below are some guidelines and best practices I always recommend and something my team follows as well.
Guidelines for Naming, Grouping, and Managing Modules
- Core Module:
- Use the CoreModule for singleton services and application-wide features, like authentication or app-wide guards.
- Import it only once in the root AppModule and never in feature modules to avoid multiple instances of services.
- Shared Module:
- Create a SharedModule for common components, directives, and pipes that will be reused across feature modules.
- Do not include services here, as this could lead to multiple instances of a service.
- Feature Modules:
- Create separate Feature Modules for each major feature or section of your app (e.g., UserModule, ProductModule).
- Group related components, services, and routes within each feature module. This keeps the codebase modular and easy to navigate.
- Lazy Load Feature Modules:
- For large, non-essential parts of your app, configure feature modules to be lazy-loaded to improve initial performance.
Modular Architecture in Large-Scale Applications
In large-scale applications, maintaining a modular architecture is key to avoiding a bloated and hard-to-maintain codebase. Here’s how you can structure your app:
- Domain-Specific Modules: Organize your app based on specific domains or business logic (e.g., UserModule, OrderModule). Each feature module should encapsulate related components and services, making them self-contained and easy to work with.
- Reusable Components: Components, directives, and pipes that are shared across multiple features should be housed in a SharedModule. This helps reduce duplication of code.
- Lazy Load for Scalability: As the app grows, lazy loading becomes a necessity. Keep non-critical modules and administrative features lazy-loaded to maintain a responsive app.
- Flat Module Structure: Avoid deep nesting of modules as it complicates routing and module management. Keep the module structure as flat as possible.
- Clear Naming Conventions: Use descriptive names for modules based on their functionality (e.g., AuthModule, DashboardModule) to make it clear what each module does. This will make it easier for future developers to understand the purpose of each module at a glance.
By following these practices, you can ensure that your Angular application remains organized, scalable, and maintainable as it grows over time.
To Sum it Up
Angular modules are instrumental for creating well-structured and scalable applications. As it helps organize your code into logical units, modules enhance maintainability, testability, and reusability. However, as we are cruising forward, a trend is emerging where Angular applications are built without NgModules.
NgModules usage will decrease as developers start using standalone components and APIs for optional NgModules. Not only will these technologies make working with Angular easier, but they will also make learning the Angular application structure easier.
Join 10,000 subscribers!
Join Our subscriber’s list and trends, especially on mobile apps development.I hereby agree to receive newsletters from Mobmaxime and acknowledge company's Privacy Policy.