From Mobile App to Desktop: Building a Progressive Web App with Flutter
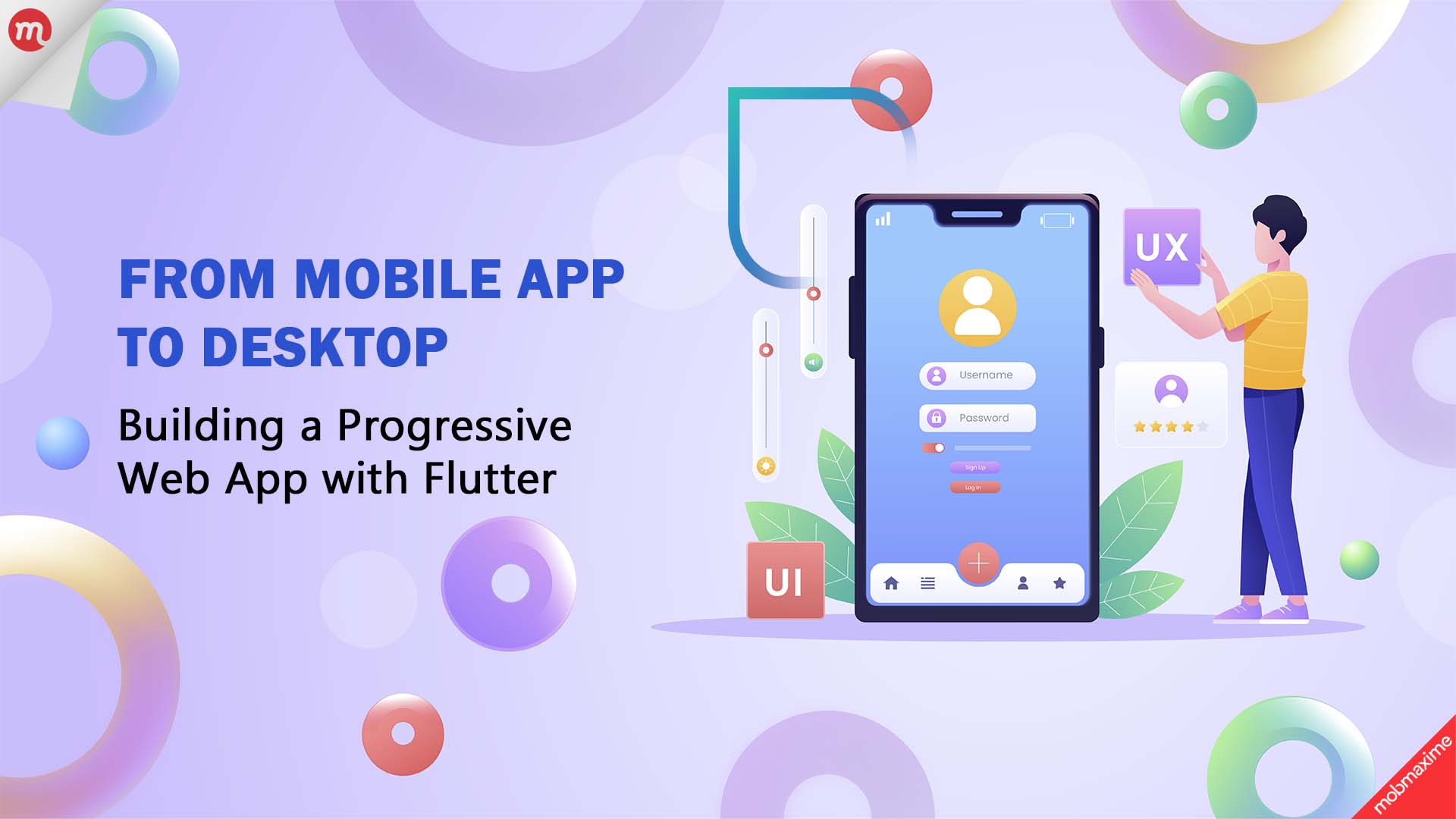
Flutter, with its single codebase, facilitates the write-once, deploy on the web, mobile, or desktop philosophy. Featuring a hot reload that speeds up the iteration and UI consistency, which ensures you build a smooth and native-like user experience for your users, Flutter is the first choice for progressive web app development.
The good news is that you can build a progressive web app with Flutter and deliver a native app-like experience. All of this is possible without the user needing to download anything from the application store.
Today we will discuss how to build a progressive web app with Flutter.
Understanding a Progressive Web Application (PWA)?
A PWA represents a mobile application built in the form of a web page accessible on any device after downloading. PWAs are held in high regard when companies need their users to have seamless and even offline accessibility without compromising on quality.
Given their resemblance to web applications, it’s easier to get confused between PWA and web apps, but they are different. PWAs are downloaded on a device, but web apps are not.
Building a PWA is easier, but when you do the same with Flutter, it gets even better. Flutter, with all its capabilities like caching, offline access, web assembly, CanvasKit, etc., helps provide a near-native experience for your customers.
Setting Up Flutter for Web Development
With Flutter, you can build progressive web applications with a single codebase, which means you can streamline the development process. However, before you begin, it’s important to set up the Flutter development environment correctly.
-
Prerequisites for Developing Progressive Web App with Flutter
Before beginning development, take care of installing or acquiring the following;
-
- Flutter SDK and get it from the official Flutter website.
- Get an IDE like VS Code or Android Studio, but check if they support Flutter development.
- You will need a browser, preferably Google Chrome, to run and debug the Flutter PWA as it develops.
- Get Flutter and Dart plugins, installing them in your IDE as it will provide a better development experience.
In addition to these tools and services, you should have basic Flutter knowledge, including understanding Flutter widgets, Flutter PWA best practices, state management, and UI development.
With everything set and installed, verify your setup by running the following: flutter doctor. If anything is missing, Flutter will guide you on fixing the same.
-
Enable Web Support in Flutter
Once set up, you need to manually toggle on web support, as it isn’t enabled by default. Use the following command to activate web support: flutter config –enable-web. Once run, confirm whether the development environment supports your web browser, run the command flutter devices, and it will generate a list of compatible browsers. Look for Chrome (Web) in the list.
-
Create a New Flutter Web Project and Running on the Web
To begin developing, you need to first create a new Flutter project using flutter create my_pwa and it will create all the necessary files required to start development. The project files will be added to the project folder cd my_pwa.
Before you work on the project, test whether it runs on Chrome using flutter run -d chrome. Running this code will launch the Flutter PWA on a new Chrome tab, and from here, you can see everything you add, change, and edit in the codebase live on the same tab.
Completing this only sets up the development environment for your Flutter PWA. Remember this is just the beginning, and in the next steps, you need to convert the app into a PWA by integration manifest, service workers, caching, and much more.
How to Create a Progressive Web App Using Flutter?
But have you ever wondered why do we need to convert a Flutter app into a PWA? Does this mean that there’s no such thing as Flutter PWA development?
Well, to some extent, it’s true. Flutter does not automatically generate a fully functional PWA. By default, A Flutter web application is a regular app without PWA features and capabilities, including;
- Installability, which means the PWA can be downloaded like a native application.
- Offline support, which allows the application to work without access to the internet.
- Service workers are required to handle caching and background tasks.
So when you are creating a Flutter web project, it doesn’t have a proper web manifest and service workers.
Converting a Flutter App into a PWA
In this section, we will discuss how to convert a Flutter application into a PWA.
-
Updating index.html for Metadata
The index.html is the entry point for your Flutter web application, and to ensure the web application runs as a PWA, you need to update it with the essential metadata. To do this, open web/index.html and add the following code script in the <head> tag.
<!– PWA Metadata –>
<meta name=”theme-color” content=”#ffffff”> <meta name=”description” content=”A Flutter Progressive Web App”> <link rel=”icon” type=”image/png” href=”icons/icon-192.png”> <link rel=”apple-touch-icon” href=”icons/icon-192.png”> <link rel=”manifest” href=”manifest.json”> |
Running this code script will help change the theme color, and the application’s description, design the app’s icons and add links to the web manifest (which we will also look at in the next step).
-
Adding a Web App Manifest (manifest.json)
The Manifest file instructs the browser about the application installation while specifying its appearance at the time of launch. Here’s how to implement this;
Start by creating a new file in the web/ folder named manifest.json followed by adding the following content;
{
“name”: “My Flutter PWA”, “short_name”: “FlutterPWA”, “description”: “A Progressive Web App built with Flutter”, “start_url”: “/”, “display”: “standalone”, “background_color”: “#ffffff”, “theme_color”: “#000000”, “icons”: [ { “src”: “icons/icon-192.png”, “sizes”: “192×192”, “type”: “image/png” }, { “src”: “icons/icon-512.png”, “sizes”: “512×512”, “type”: “image/png” } ] } |
Running this code script as you convert the mobile app to PWA Flutter will set the name and short_name of the application when installed. It will define the starting page of the application via the start_url command and also ensure the application launches without the browser UI, just like a native application.
-
Using Service Workers for Caching
Service workers, with reference to Flutter PWA development, is a background script that connects the web application with the network. As a result, the PWA you build can have features like offline accessibility, caching network requests, push notifications, and background synchronisation.
To implement this, you need to run flutter build web and Flutter will automatically generate service worker flutter_service_worker.js in build/web/. Now, you must check if caching works as intended, and for this, ensure web/index.html contains the following code script.
<script>
if (‘serviceWorker’ in navigator) { window.addEventListener(‘load’, () => { navigator.serviceWorker.register(‘flutter_service_worker.js’); }); } </script> |
Running the code will not only check caching but register the service worker to cache assets and present them online when asked. Next it also ensures the application loads faster, especially after the first visit.
-
Testing Offline Functionality
Now that you have completed the steps above let’s test Flutter PWA to know whether it works without an internet connection or not.
-
- Run the Flutter application in Chrome using the command flutter run -d chrome.
- In Google Chrome, open Developer Tools and click on Inspect.
- In Inspect, go to the Application tab and find Service Workers.
- From here check offline mode under network conditions and reload the application to check if it loads.
With these four steps, you can convert the mobile app to PWA Flutter and give it installability and offline support. Now, what’s required is for you to optimize the app’s performance and deploy it on Firebase, Vercel, or Netlify, or any other solution according to your requirements.
Performance Enhancements in Flutter PWA
The general approach to enhance the web performance of a Flutter PWA is minimizing the unnecessary widget rebuilds with the const and using efficient data structures for lists. In addition to these things, which I guess you already know and can implement easily, here’s what I recommend you do.
-
Understand the Difference Between CanvasKit and HTML Renderer
With Flutter, you can use two rendering backends: CanvasKit and HTML.
-
- CanvasKit: This backend renderer uses WebAssembly (Wasm) to render graphics using Skia. CanvasKit is recommended because Skia is the default engine for native Flutter applications. Using CanvasKit, you can ensure better graphical performance and it’s ideal for applications that need rich animations.
- HTML: HTML renderer is CSS and DOM-based, and it uses the standard HTML, CSS, and Canvas API to render UI. In addition to this, HTML renderer produces smaller bundle sizes of UI elements, but it does lack some advanced effects. Hence, only use HTML renderer if the application is meant for low-end devices that run on slower networks.
You will have to specify the renderer you will use for enhancing PWA’s aesthetics and performance. If it’s not specified with either the command flutter build web –web-renderer=canvaskit or flutter build web –web-renderer=html, Flutter will automatically pick the best renderer based on the browser and device.
-
Lazy Loading Assets
Flutter has a default setting to load all assets upfront, which increases the initial load time. But there’s a way to bypass this by using Lazy Loading, as it helps load assets only when required. Here’s how to implement Lazy Loading;
With Lazy Loading, instead of preloading all the images, you can program Flutter to load them dynamically.
Image.network(
‘https://example.com/large-image.png’, loadingBuilder: (context, child, loadingProgress) { if (loadingProgress == null) return child; return Center(child: CircularProgressIndicator()); }, ); |
Implementing this code script means you can reduce the initial application load time and load images only when needed. Moreover, this ensures the application works seamlessly, even in slower-performing networks.
-
Work on Reducing the Bundle Size
I guess you already know that Flutter compiles everything into JavaScript, which means the final bundle size can be large and reducing it is your only option if you need to have excellent performance.
So how can you achieve that?
-
- Remove unused fonts, assets, and packages from the application. Check pubspec.yaml to get rid of all such unnecessary dependencies.
- Another thing which Flutter does automatically but you need to verify is Minfy and Tree-shake code using flutter build web –release. This will remove the unused code from the application and also optimize it.
- Lastly, in this step, use Gzip and Brotli Compression to set up your hosting platform. You can choose between Firebase, Netlify, and Vercel. These hosting platforms are suggested because they enable Gzip or Brotli to reduce the file’s size.
Deployment of Flutter PWA
After covering Flutter PWA development, let’s move on to deploying the application you have just built. Flutter PWA provides built-in web support for deploying the applications, which makes the entire process easier. There are only a few extra steps you need to take once the application is built for deployment.
-
Have a Production-Ready Build
Prior to deployment, your application should be ready for production, and you can ensure this by using flutter build web. It will generate the necessary static files. But you must also test the application locally before deploying.
Use the code script – cd build/web python3 -m http.server 8000. Once done, open http://localhost:8000 in a browser.
-
Different Hosting Options
As we have said before, there are different hosting options for progressive web apps with Flutter, including Firebase, Vercel, and GitHub pages. Select the relevant hosting option according to your requirements.
All three hosting platforms above have HTTPS inbuilt, but if you are taking the self-hosting option, ensure to obtain an SSL certificate for deploying the application.
Conclusion
Flutter lets you build high-performance PWAs with a single codebase. Using Flutter, you can build PWAs that represent the best of web and mobile experiences. Flutter is the preferred solution to build fast, installable, and offline-capable PWAs.
However, when building Flutter PWAs, you will need to understand its setup requirements and more importantly, the process to convert the mobile app to PWA Flutter. With continuous advancements, the Flutter team is continuously improving web rendering and effectively reducing the bundle size so that developers like yourself can enhance loading speed.
If that’s not all, more native-like web experience features like push notifications and file handling are also expected in future Flutter updates. So stay tuned to know about these updates and if you need help with Flutter PWA development, get in touch with Mobmaxime.
We can help!
Join 10,000 subscribers!
Join Our subscriber’s list and trends, especially on mobile apps development. [mc4wp_form id=6800]I hereby agree to receive newsletters from Mobmaxime and acknowledge company's Privacy Policy.