Offline-first Flutter Apps: Using Hive & SQLite for Local Storage
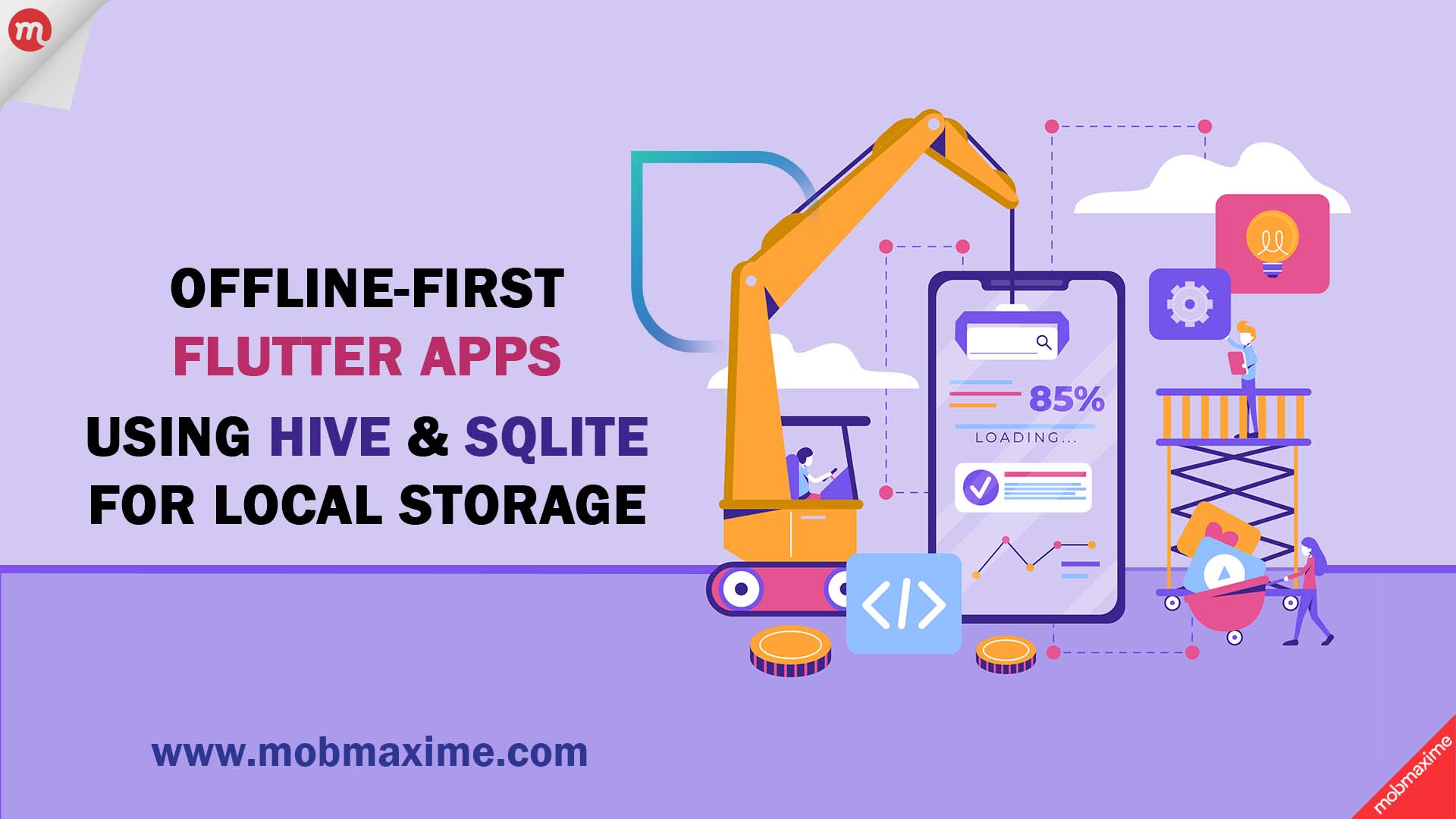
In today’s digital world, offline-first app development is crucial for providing users with a seamless experience, even in low or no network connectivity situations. Flutter, being one of the most popular cross-platform frameworks, offers excellent solutions for local storage using Hive and SQLite. These databases help in Flutter offline data synchronization, ensuring that applications remain functional without a continuous internet connection.
In this blog, we will explore how to implement Flutter local database solutions using Hive and SQLite, their advantages, and when to use each for your Flutter applications.
Must Read: Want to Know About Flutter? This One Guide is Enough!
Why Offline-First Development is Important
An offline-first app ensures that users can continue using an application without an active internet connection. This is particularly useful for:
- Remote locations with poor connectivity
- Applications that handle critical data such as finance, healthcare, and productivity tools
- Enhancing user experience by reducing dependency on network latency
By implementing offline-first app development, businesses can offer a more reliable and engaging user experience that keeps users coming back.
Choosing the Right Local Storage: Hive vs. SQLite
Both Hive and SQLite are great local storage solutions in Flutter, but they serve different purposes:
Feature | Hive | SQLite |
---|---|---|
Best for | Key-value storage, lightweight apps | Relational data, structured queries |
Speed | High performance, optimized for Flutter | Slower than Hive but ideal for relational data |
Querying | No SQL, works with objects | SQL-based querying, supports relationships |
Offline support | Excellent | Excellent |
Use case | Caching, settings, small data | Complex databases, relational data management |
Implementing Hive for Offline-First Flutter Apps
Hive is a lightweight and fast Flutter local database, making it an excellent choice for applications that need quick access to key-value pairs.
Steps to Implement Hive:
- Add Dependencies
dependencies:
hive: ^2.2.3
hive_flutter: ^1.1.0
- Initialize Hive in
main.dart
:
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Hive.initFlutter();
runApp(MyApp());
}
- Create and Use a Hive Box:
var box = await Hive.openBox(‘settings’);
box.put(‘theme’, ‘dark’);
print(box.get(‘theme’)); // Output: dark
Implementing SQLite for Offline-First Flutter Apps
For structured and relational data, SQLite is the preferred choice. It supports complex queries, relationships, and transactions.
Steps to Implement SQLite:
- Add Dependencies
dependencies:
sqflite: ^2.2.0
- Create Database and Tables:
Database database = await openDatabase( 'my_database.db', version: 1, onCreate: (db, version) { return db.execute( 'CREATE TABLE users(id INTEGER PRIMARY KEY, name TEXT, age INTEGER)' ); }, );
- Insert and Retrieve Data:
await database.insert(
‘users’,
{‘id’: 1, ‘name’: ‘John’, ‘age’: 30},
);
List<Map<String, dynamic>> users = await database.query(‘users’);
print(users);
Implementing Flutter Offline Data Synchronization
To ensure data consistency between local storage and a remote database, follow these best practices:
- Use timestamps to track data updates
- Sync data in the background when the internet is available
- Resolve conflicts by implementing a conflict resolution strategy
- Use Firebase or REST APIs to push and fetch changes efficiently
When to Use Hive vs. SQLite in Your Flutter App
Use Hive when: ✅ You need fast key-value storage for caching or small-scale data storage ✅ Your app does not require complex relationships between data ✅ You prioritize performance over complex queries
Use SQLite when: ✅ Your app requires relational data management ✅ You need advanced querying capabilities ✅ You want ACID compliance for data consistency
Conclusion
Building an offline-first app with Hive and SQLite in Flutter ensures that your users get the best experience, even without an internet connection. Depending on your application needs, you can choose between Hive for lightweight key-value storage or SQLite for structured relational data.
Looking to develop a high-performance Flutter app with offline capabilities? A top Flutter app development company can help you design and implement robust local storage solutions tailored to your business needs.
Contact us today to build your next Flutter-powered offline-first application!
Join 10,000 subscribers!
Join Our subscriber’s list and trends, especially on mobile apps development. [mc4wp_form id=6800]I hereby agree to receive newsletters from Mobmaxime and acknowledge company's Privacy Policy.