Flutter for Machine Learning: Integrating AI into Your Mobile Apps
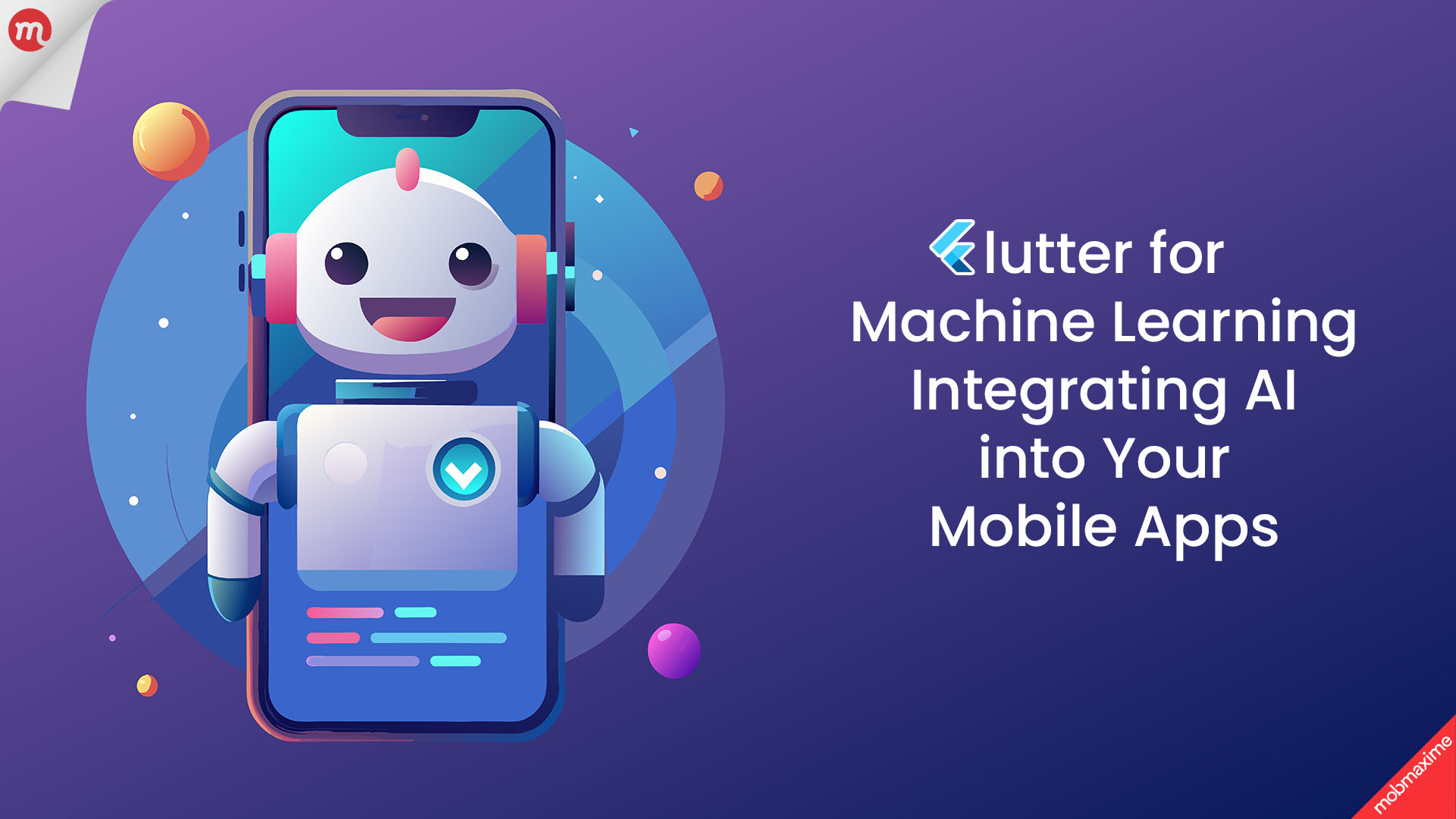
Flutter is one of the most effective platforms for integrating Artificial Intelligence (AI) and Machine Learning (ML). A cross-platform technology, Flutter has experienced a growing ecosystem compatible with AI/ML libraries and tools. This ease of integration makes Flutter a foremost choice for developers to include advanced technologies into an application.
I have spearheaded my team several times in adding one or the other AI/ML-related functionality into a client’s application. Using my expertise and experience in this field, I’ll go through the process of integrating AI into the Flutter app.
Why Only Flutter for AI/ML Integration?
Flutter is a cross-platform framework, but you already know that. What I want to highlight is that this cross-platform compatibility of Flutter makes it a better choice than choosing a native technology like Swift, Kotlin, etc., for AI/ML integration. This means even with adding complex AI/ML features, which takes time to add, Flutter apps will have a shorter time to market.
Furthermore, when compared to React Native and Xamarin, Flutter has outperformed both due to its direct compilation of native code and UI customization capabilities. Moreover, the hot reload feature in Flutter is exceptionally useful here as it accelerates development and testing cycles, which are essential to implement the perfect AI/ML models.
Reasons to Integrate AI in Flutter
Integrating AI into the Flutter app means enhancing user experience and adding personalization to the same using chatbots, voice assistants, recommendations, etc. Moreover, AI and ML allow for better decision-making, enabling businesses to innovate and stay ahead of the competition.
With AI and ML, Computer Vision (CV) is also used to create smart applications to further improve the app’s functionality. Specifically, CV is used to recognize objects and faces while performing other image-based functions.
So, advanced features like image recognition, natural language processing, personalized recommendations among others fit seamlessly into an application because of this integration.
Role of Dependencies in AI/ML Integration in Flutter App
Dependencies represent the backbone of AI/ML integration in Flutter app development. These dependencies provide the essential tools and libraries you will need to build interaction between AI models and process data. Here are a few core functionalities dependencies can take care of;
- AI Framework Integration: TensorFlow Lite (tflite) or ML Kit (firebase_ml_vision) packages provide the fundamental APIs developers need to load, run, and interpret AI models. In addition to this, some dependencies we use for development provide platform-specific implementations.
- Handling Data: Dependencies are used for libraries like image or image_picker to capture, load, and preprocess images for AI models. Then we have been using them for data structures and handling numerical data the AI models you include in the application need.
- Model Deployment: During model deployment, dependencies are used for asset management, where packages like flutter/assets assist with managing AI/ML model files and additional resources. Similarly, for cloud-based AI services, http or other platform specific cloud SDKs are used.
- UI Integration: Visualization libraries like charts, graphs, and other data visualization assets are used to display AI model outputs effectively. At the same time, dependencies for the app’s UI components are also essential to build user interfaces and their interactions with AI features.
How to Integrate AI into Flutter App?
It’s a step-by-step process of integrating AI into the Flutter app, at the end of which you will build a solution with seamless advanced functionality and enhanced user experience.
-
Define Use Case and Requirements
Begin by identifying the specific AI functionalities you need to integrate into the Flutter application. If you are building the application for a client, it’s best to ask them about the functionality they wish to add.
Some of the most added AI functionalities are image recognition, predictive analytics, NLP, etc. For each feature or functionality, define the requirements like data inputs and expected outputs. This will help you define how adding AI will enhance user experience and application functionality.
-
Choose the AI Framework or Library
For adding AI features into the Flutter application, you need to choose a framework or library. Among the best ones, there are TensorFlow Lite, ML Kit, and IBM Watson.
Instead of choosing a framework or library, you can also use pre-trained AI models. These models have a variety of functionalities you will need to build AI-enabled Flutter applications.
However, pre-trained models aren’t as customizable as you want them to be. If you want to add specific functionalities and train your models, you will need to custom-build them.
-
Work on the Development Environment
The specifics of this step can vary according to the AI library you have chosen to build the application. But here are the general steps you need to follow;
-
- Install Flutter on the system and set up the IDE, which must have the Flutter plugging.
- Install the required dependencies by adding necessary Flutter packages to the pubspec.yaml file. Through this file, you can add features specific to the chosen platform (iOS, Android), UI components, and general purpose utilities. The specific package you have to add will depend on the AI/ML library you are using for the project.
-
Integrate the AI Framework
You will find the installation instructions for your chosen framework. Following them, install the SDKs or required libraries and configure the environment variables along with project settings. After completing this part, add platform-specific code, which will again depend on the platforms for which you are developing the application.
The process does not stop here. All of the next steps depend on the framework and platform you have chosen. I’ll take you through the integration steps of three main AI/ML frameworks and libraries;
-
ML Kit
Built by AI and ML experts at Google, when working with ML Kit, you will realize its potential. Bringing forth Google’s machine learning expertise to the app development environment, ML Kit is a powerful and easy-to-use framework. You will get ready-to-use APIs for the most common use cases like text and image recognition, detecting faces, identifying landmarks, barcode scanning, etc.
To implement its functionality into a Flutter machine-learning app, use the following code;
import ‘package:firebase_ml_vision/firebase_ml_vision.dart’; void detectLabels(Image image) async {
final ImageLabeler labeler = FirebaseVision.instance.imageLabeler();
final List<ImageLabel> labels = await labeler.processImage(image);
for (ImageLabel label in labels) {
final String text = label.text;
final double confidence = label.confidence;
print(‘Label: $text, Confidence: $confidence’);
}
}
-
IBM Watson
Boasting a variety of AI services, IBM Watson is yet another amazing framework for integrating AI into Flutter app. The services include Language Translator, Text-to-Speech, Visual recognition, among others. Built upon the latest innovations, IBM Watson is making it easier for developers to add AI/ML capabilities into an application.
To set up IBM Watson, you will need to create an IBM Cloud account. Once done, create an instance of the specific service you want to include in the application. After this, add the flutter_ibm_watson package to the pubspec.yaml file.
dependencies: flutter_ibm_watson: ^0.0.7
Once the package is sourced, use the following code script to use IBM Watson’s services;
import ‘package:flutter_ibm_watson/flutter_ibm_watson.dart’; void translateText(String text) async {
IamOptions options = await IamOptions(iamApiKey: “api-key”, url: “url”);
LanguageTranslator service = new LanguageTranslator(iamOptions: options);
TranslationResult result = await service.translate(
text: text,
source: ‘en’,
target: ‘es’,
);
print(result);
}
-
TensorFlow Lite
Another one of the AI/ML frameworks by Google, TensorFlow Lite allows developers to run the in-built models on mobile, embedded, and IoT devices. This comes with on-device machine learning inference and a low latency rate. Specially designed for mobile devices, you can train this framework to train and deploy machine learning in Flutter applications.
You follow the code script to integrate TensorFlow Lite into your Flutter app environment;
void detectLabels(Image image) async { await loadModel();
List<double> input = await imageToInput(image);
List<Map<String, dynamic>> output = await runModel(input);
// Process output to extract labels and confidence scores
for (Map<String, dynamic> label in output) {
String text = label[‘label’];
double confidence = label[‘confidence’];
print(‘Label: $text, Confidence: $confidence’);
}
}
-
-
Implementing AI Functionality in Flutter App Development
The implementation part begins with UI integration where you design Flutter widgets with AI features. These are features like adding a camera widget for image-based AI tasks or text input fields for NLP-based functionalities. Similarly, you can add features for output visualization and loading indicators.
With this done, define the data pipelines, including tasks like data preprocessing where you will implement functions to preprocess input data. This data input will depend on the AI model requirements.
Next, work on model interaction where you have to define methods to pass the preprocessed data to the AI model. Plus create functions to interpret and process the AI model’s output to extract relevant information.
Three Ways to Interact with AI Model
- Model Loading: Integrate app code to load the AI model into local storage or a remote source memory.
- Model Inference: This includes implementing functions for executing AI models on preprocessed data and obtaining the predictions.
- Model Caching: As you consider caching model results, use them to improve application performance and reduce latency.
import ‘package:flutter/material.dart’; import ‘package:tflite/tflite.dart’;
import ‘dart:typed_data’;
import ‘package:image_picker/image_picker.dart’;
class ImageClassifier extends StatefulWidget {
@override
_ImageClassifierState createState() => _ImageClassifierState();
}
class _ImageClassifierState extends State<ImageClassifier> {
File? _image;
String? _result;
Future<void> _loadModel() async {
// Load TensorFlow Lite model
}
Future<void> _classifyImage() async {
// Preprocess image, run inference, and update _result
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text(‘Image Classifier’)),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
_image != null ? Image.file(_image!) : Text(‘No image selected’),
ElevatedButton(
onPressed: () async {
final pickedFile = await ImagePicker().pickImage(source: ImageSource.gallery);
if (pickedFile != null) {
setState(() {
_image = File(pickedFile.path);
});
_classifyImage();
}
},
child: Text(‘Choose Image’),
),
Text(_result ?? ”),
],
),
),
);
}
}
The code script you see above is written in Dart programming language for an image classification application.
-
Testing and Validating the Flutter AI Application
When you build a Flutter machine learning app, the scale and size of the application increase naturally. Due to the complex functionalities added to the app, it will need comprehensive Flutter testing to ensure performance and reliability.
-
- Device and Platform Compatibility: Test the application on various emulators and simulators for iOS and Android. In addition to these, also test the app on real devices that too with different screen sizes and resolutions and hardware specifications.
- User Scenarios and Use Cases: You will test the application for typical usage by default. But to this testing process, add extreme conditions and error handling while including some edge cases (unusual or unexpected user actions).
- Performance Testing: In performance testing, analyze the app’s response time, which is the time taken for the AI model to process given input and generate output. Plus, use resource utilization to assess CPU, memory, and battery usage.
- AI/ML Specific Validation: For AI-specific application testing, we use some specific tools for model evaluation, data validation, and debugging. Other tools will also work but these specific ones have in-built structures to help you test AI-enabled applications easily.
Challenges in Integrating AI in Flutter Applications
Integrating AI into the Flutter app is promising. But you can come across several challenges in the process. While the specific challenges can depend on your development approach, here are some of the most common challenges developers face, along with the issues we came across.
-
Model Selection
Choosing the right AI model for all the tasks, given their specificity, can be complex. With a wide range of available options and their characteristics, choosing one can be difficult.
-
SDK Compatibility and Support
Several compatibility issues can arise in the integration process. Different SDKs and frameworks have different compatibility requirements. So, TensorFlow Lite or ML Kit, among other frameworks, have different compatibility issues and support for certain features and mobile platforms.
-
Optimizing App Performance
Mobile devices vary in various aspects, and one of them is the optimization strategies to maintain app responsiveness and battery consumption. Taking into account these differences, you need to balance the AI model’s complexity and performance requirements while ensuring it does not affect the device’s capabilities.
-
Integration Complexities
AI and ML are intricate technologies that are added to an application by following intricate workflows. From data preprocessing to model loading, image processing, and model deployment, among others, it demands expertise. So, if you are planning to hire Flutter app developers, ensure they are well-experienced and experts at what they do.
Conclusion
Integration of machine learning and artificial intelligence in Flutter helps build smart and modern applications. Leveraging their capabilities, you can create more intuitive and engaging applications to improve user experience. However success will only come when you are using the right tools and experts for the task.
At Mobmaxime, we have everything you need to leverage Futter’s powerhouse of features and functionalities. A top Flutter app development company, our in-house Flutter experts work with AI/ML experts to build a common understanding of your requirements and work on the deliverables.
Are you interested in a small discussion for your next Flutter project? Book a call with us to open up your business to a world of new opportunities and possibilities.
Join 10,000 subscribers!
Join Our subscriber’s list and trends, especially on mobile apps development.I hereby agree to receive newsletters from Mobmaxime and acknowledge company's Privacy Policy.