Implementing Custom Widgets in Flutter: Unleashing Creativity and Efficiency
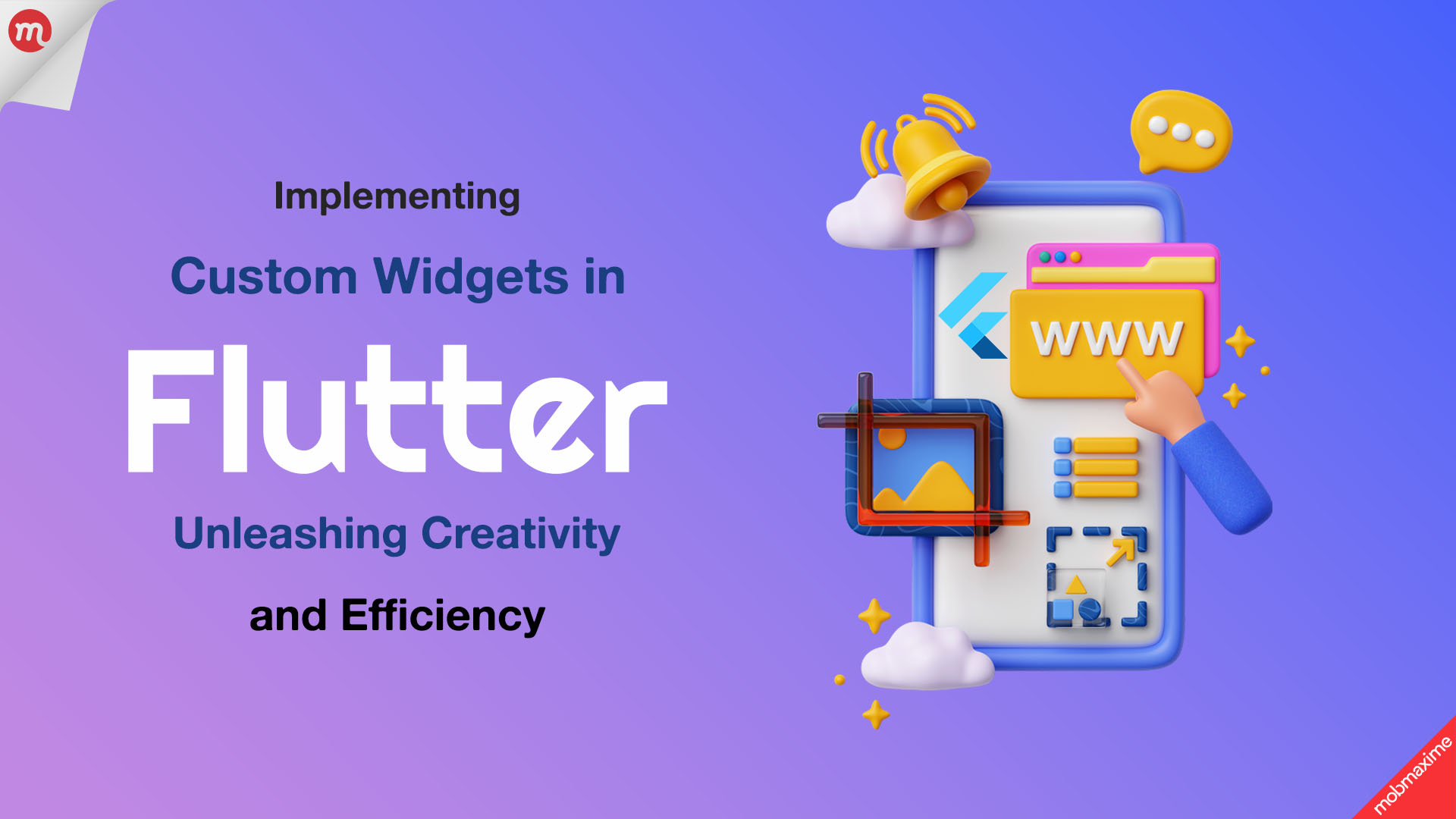
Flutter boasts a rich library of pre-built widgets, which aid in design and development. However, there are times when you need more control or specific functionality to build applications.
This is where custom widgets in Flutter come into play. Implementing them means you can create tailored components to match your app’s unique design and functionality requirements. As a result, you can ensure the app’s scalability and reusability across your codebase.
To implement custom widgets, start by identifying common patterns or unique UI elements in the application. Then, break them into smaller and reusable widgets. Implement them as either StatelessWidget or StatefulWidget, depending on your need to maintain an internal state.
Role of Custom Widgets in the Flutter Widget Tree
Everything’s a widget when it comes to Flutter. The UI of a Flutter application is built by combining the hierarchy of these widgets, which we call the widget tree. Now, as we know, custom widgets are a key part of this structure.
Why? They allow you to break down your UI into manageable, reusable components.
Custom widgets can be used at any level of the tree, from individual buttons to entire screens. Through this process, they help create clean, modular, and maintainable code. The purpose of a widget tree is to ensure your custom widgets interact seamlessly with built-in widgets, effectively making the app’s UI structure more organized and efficient.
Here’s how I recommend you use widget customization in Flutter. For complex UIs, break down the interface into logical chunks through custom widgets. This implies that instead of integrating multiple built-in widgets in a large tree, a custom widget should be used that includes all the common UI patterns. As a result, you can reduce clutter in the main widget tree while making the codebase easier to maintain and scale.
Refactoring Custom Widgets to Write Clean and Maintainable Code
In Flutter, refactoring is important as it helps ensure that your code remains manageable even when the application’s complexity grows. Refactoring custom widgets means restructuring existing code without altering the external behavior to make it more efficient and readable.
Breaking Down Complex Widgets
- Large, monolithic widgets can be difficult to manage, test, and debug; hence, refactoring them into smaller, self-contained widgets improves readability and reusability.
Rather than embedding all UI logic in one large widget, break it down into smaller custom widgets, such as CustomButton, CustomCard, or CustomFormField. This way, each widget serves a single purpose and can be reused in multiple parts of your app.
Reusing Common Logic
- With repeated patterns in the widget tree, refactor those patterns into reusable widgets to eliminate code duplication, making updates and bug fixes easier. For instance, when you use a specific button style throughout the app. Refactoring it into a reusable CustomStyledButton widget lets you change the style in one place rather than multiple files.
Managing State Efficiently
- By implementing refactoring, you can also manage state effectively and organize how widgets communicate and share data. You can achieve this by separating state management logic from UI code, making it easier to maintain. A good example would be moving complex state management logic into a dedicated service or model class and using widgets strictly for rendering the UI. This separation of concerns keeps your code modular and reduces clutter in your widget classes.
Stateless Custom Widgets in Flutter
Having worked in the industry for 20+ years, I recommend you use this when the widget doesn’t need to manage any internal state. Hence, they are called static widgets that remain changed once rendered.
Additionally, custom stateless widgets are important when adding buttons, static headers, or icons in the application. Moreover, their implementation is simple. All you have to do is define the widget’s build method to initiate a return of the UI elements.
Implementing Custom Widgets in Flutter | StatelessWidget
For implementing a custom StatelessWidget, I prefer creating reusable components that won’t need to manage the app’s internal state.
Codescript for adding Reusable Button
import ‘package:flutter/material.dart’; class CustomButton extends StatelessWidget { final String label; final VoidCallback onPressed; final Color color; final TextStyle textStyle; const CustomButton({ Key? key, required this.label, required this.onPressed, this.color = Colors.blue, this.textStyle = const TextStyle(color: Colors.white, fontSize: 16), }) : super(key: key); @override Widget build(BuildContext context) { return ElevatedButton( onPressed: onPressed, style: ElevatedButton.styleFrom(primary: color), child: Text(label, style: textStyle), ); } } |
Here’s what’s happening in the code;
- Properties: The widget you are adding accepts customizable parameters like label, onPressed, color, and textStyle. Following this ensures you can use the button in various places simply by changing appearances and behaviors.
- Customization: By exposing properties like color and textStyle, you allow other developers working on the application to tailor the button and fit it to different parts of the app while maintaining a consistent structure.
- Build method: At the base of the widget we have used Flutter’s ElevatedButton, and the customization options to the widget are applied through styleFrom and Text.
Because we have created custom stateless widgets like the button examples shown above, it reduces redundancy across the projects while helping achieve performance optimization in Flutter.
Moreover, rather than defining a button every time, now we can reuse the CustomButton widget, to ensure uniformity across the application and reduce potential for errors. It makes the UI more consistent and my codebase easier to maintain.
Implementing Custom Widgets in Flutter | StatefulWidget
This custom widget in Flutter is used when you need to handle dynamic data or implement UI changes. These widgets help maintain state and can even rebuild themselves when the predefined state changes. Custom stateful widgets are useful for interactive elements like forms, toggles, or counters.
Since we implement interactive elements in Flutter through Stateful widgets, here’s an example of a simple counter widget that responds to user input by increasing or decreasing the count.
Codescript for Custom Counter
import ‘package:flutter/material.dart’; class CustomCounter extends StatefulWidget { @override _CustomCounterState createState() => _CustomCounterState(); } class _CustomCounterState extends State<CustomCounter> { int _count = 0; void _increment() { setState(() { _count++; }); } void _decrement() { setState(() { _count–; }); } @override Widget build(BuildContext context) { return Row( mainAxisAlignment: MainAxisAlignment.center, children: [ IconButton( onPressed: _decrement, icon: Icon(Icons.remove), ), Text( ‘$_count’, style: TextStyle(fontSize: 24), ), IconButton( onPressed: _increment, icon: Icon(Icons.add), ), ], ); } } |
Here’s what is happening in the above code script;
- State management: The widget code you see above uses an integer _count to record and store the current count. This state is modified through the _increment and _decrement functions, which we denote as setState to trigger a UI update.
- Interactivity: The IconButton widgets allow the user to increase or decrease the count by triggering state changes.
- Build method: The UI consists of a row containing two buttons and a text widget that displays the current count.
Using StatefulWidget for interactive components in the application, like counters, forms, or switches, streamlines how a developer manages UI changes. Integrating the logic within the widget it’s easier to avoid messy state handling anywhere else in the app. As a result, this makes the application you are building clear and leads to performance optimization in Flutter.
Must Read: Exploring Flutter: Essential Packages and Plugins for Developers
Best Flutter Widget Customization Techniques
The purpose of using widgets in Flutter is to make the components modular, maintainable, and scalable across the application. Here are the strategies to implement these practices;
Modular Design
To build an application with modular design, break down your user interface into smaller components that serve a specific purpose. Here, every widget you add must be self-contained and manage a unique function, effectively making it easier to reuse across different parts of the app.
For instance, if you have a list of items, you can create a reusable CustomListItem widget instead of repeating the code every time. This widget can accept parameters like text, icon, or image, and you can use it across various screens where similar item structures are required.
Avoid Overcomplicating Widgets
Another way widgets can help in enhancing Flutter app performance is by keeping the widgets simple. In case the widget you have added handles too many components, break it down into smaller widgets. Not only will this make development and management easier, but simpler widgets are also easier to test, debug, and reuse.
Use Constructor Parameters for Flexibility
Use constructor parameters to expose application-related properties. Doing so will ensure that your widgets are reusable with different configurations, too, without having to change their internal logic.
Composition Over Inheritance
There’s a better way than inheriting from other widgets, which is composing widgets by combining smaller ones into a larger component. This will keep your code cleaner and help you avoid the complexities of inheritance. This works well when you are building a complex card UI and composing it using CustomImage, CustomText, and CustomButton widgets rather than creating an entire card widget from scratch.
Enhancing Custom Widgets with InheritedWidget and Provider
When using InheritedWidget for data sharing, you can share data between widgets in the widget tree without passing it through constructors. Doing so will make the development process easier to manage, especially the state or context that needs to be accessed by multiple child widgets.
To implement this, start by creating a custom InheritedWidget to encapsulate the subtree requiring access to shared data. In addition to this, inside the child widgets, use InheritedWidget.of(context) to access the data.
For instance, you can use InheritedWidget to share theme settings on multiple screens. This is useful as one of the ways to implement custom widgets in Flutter, as you can access it directly in each widget instead of passing the theme data through each widget’s constructor, making the code cleaner.
Implementing Provider for State Management
Provider in Flutter is built on top of InheritedWidget, and it facilitates an efficient way to manage and access state. The purpose of Provider is to let you easily manage state across the widget tree, providing a more robust and scalable solution for larger applications.
To implement this, start by wrapping your widget tree in a ChangeNotifierProvider or Provider, exposing your data or state model. Child widgets can then use Provider.of<T>(context) to access the shared data or state.
As the best Flutter app development company we have successfully used Provider in a large e-commerce app which requires multiple widgets to access the user’s shopping cart data.
So, instead of passing the data via various widget constructors, we use Provider to make it available throughout the app, making state management simpler and scalable as the app grows.
Custom Painting and Some Advanced Custom Widgets in Flutter Practices
CustomPainter is a powerful tool we use for advanced Flutter development. It allows the creation of unique, pixel-perfect designs and shapes within the application. So, whether it’s about drawing complex shapes or animating the UI, CustomPainter gives you absolute control over the canvas.
With CustomPainter, you can define how widgets are painted; for instance, you can instruct it to override the paint method. This gives you access to the low-level drawing APIs in Flutter, enabling you to do more than what’s achievable with basic widgets and creating custom visual effects, graphics, and animations.
Code script for adding Custom Progress Bar
import ‘package:flutter/material.dart’; import ‘dart:math’; class CircularProgressBar extends StatelessWidget { final double progress; const CircularProgressBar({Key? key, required this.progress}) : super(key: key); @override Widget build(BuildContext context) { return CustomPaint( size: Size(100, 100), painter: ProgressBarPainter(progress), ); } } class ProgressBarPainter extends CustomPainter { final double progress; ProgressBarPainter(this.progress); @override void paint(Canvas canvas, Size size) { final Paint paint = Paint() ..strokeWidth = 10 ..style = PaintingStyle.stroke ..color = Colors.blue; final Paint backgroundPaint = Paint() ..strokeWidth = 10 ..style = PaintingStyle.stroke ..color = Colors.grey; final center = Offset(size.width / 2, size.height / 2); final radius = min(size.width / 2, size.height / 2) – 10; // Draw background circle canvas.drawCircle(center, radius, backgroundPaint); // Draw progress arc double sweepAngle = 2 * pi * (progress / 100); canvas.drawArc( Rect.fromCircle(center: center, radius: radius), -pi / 2, // Start from the top sweepAngle, false, paint, ); } @override bool shouldRepaint(CustomPainter oldDelegate) { return true; // Repaint whenever progress changes } } |
Using CustomPainter, we have been creating highly customized UI elements and components that let us push the boundaries of Flutter’s design capabilities. By doing so, you can add bespoke elements like animated progress bars, intricate loaders, and custom shapes, giving your application a distinctive visual appeal that pre-built widgets fail to achieve.
To Sum it Up
Custom widgets are essential for maintaining Flutter’s flexibility, ensuring developers can build and maintain unique, scalable, and efficient UIs. Whether it’s about creating reusable components, managing state with tools like Provider, or pushing the limits with custom painting, knowing how to use custom widget implementation is key to delivering standout apps.
If you’re looking to develop a Flutter app that leverages advanced UI features, Mobmaxime can help you bring your ideas to life. Leverage our expertise in Flutter development, as we create tailored, high-performance applications to meet your specific business needs.
Contact us today to discuss how we can help build your next cutting-edge Flutter application.
Join 10,000 subscribers!
Join Our subscriber’s list and trends, especially on mobile apps development.I hereby agree to receive newsletters from Mobmaxime and acknowledge company's Privacy Policy.