Secret Flutter Features You Didn’t Know Existed (But Should!)
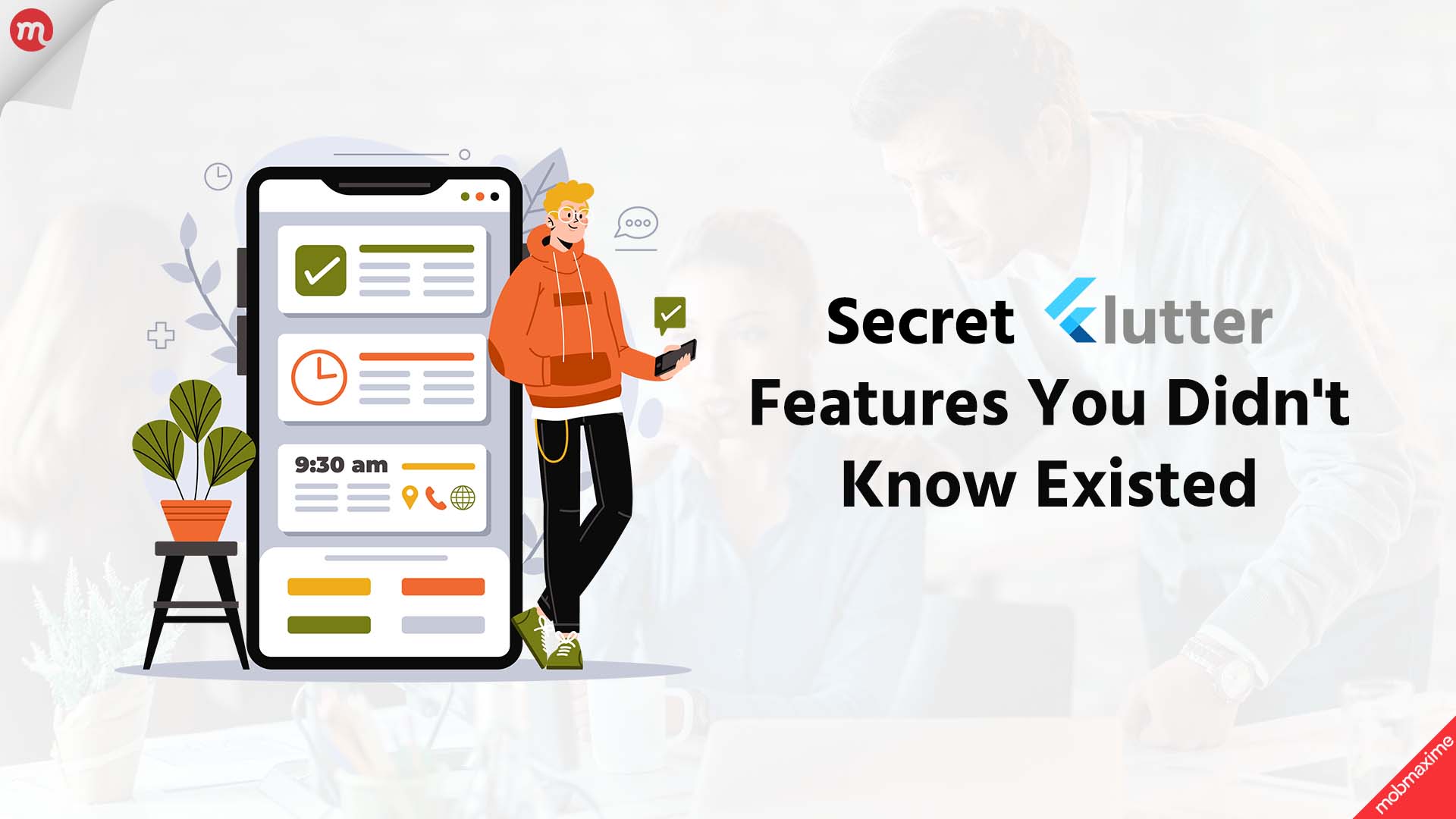
Flutter, the open-source UI software development toolkit by Google, has been making waves in the development community for its efficiency, flexibility, and cross-platform capabilities. While many developers are familiar with its core features, there are several lesser-known functionalities that can significantly enhance your development experience. In this blog, we will explore some secret Flutter features that you probably didn’t know existed, but definitely should!
1. Hot Reload and Hot Restart
-
Hot Reload
Most developers are familiar with Hot Reload, a feature that allows you to see the changes made to your code almost instantly without restarting the entire app. However, what you might not know is the extent of its capabilities. Hot Reload preserves the state of your app, making it incredibly useful for debugging and experimenting with your UI.
-
Hot Restart
On the other hand, Hot Restart resets the app state but is still faster than a full restart. It’s perfect for situations where you need to see how your changes affect the initial state of your app.
2. State Restoration
Flutter’s state restoration allows your app to save and restore its state across sessions. This feature is essential for improving user experience, especially in apps that are frequently interrupted by other tasks. To implement state restoration, you can use the RestorationMixin
and the RestorationManager
.
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
} class _MyAppState extends State<MyApp> with RestorationMixin {
// Implementation details
}
3. Flutter DevTools
Flutter DevTools is a suite of performance and debugging tools specifically designed for Flutter. Beyond the basics of debugging, you can use it to:
- Analyze memory usage
- Inspect the widget tree
- Profile your application
- Monitor network traffic
These tools provide deep insights into your app’s performance, helping you to optimize and troubleshoot more effectively.
4. Custom Painter
The CustomPainter
class allows you to create custom graphics and animations that are not possible with the standard widget set. With CustomPainter
, you can draw directly on the canvas and create complex shapes, paths, and animations.
class MyPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
// Drawing code here
}@override
bool shouldRepaint(covariant CustomPainter oldDelegate) {
return false;
}
}
5. In-App Purchases
Monetizing your app is crucial, and Flutter provides a seamless way to integrate in-app purchases. The in_app_purchase
package supports both iOS and Android, allowing you to manage products, handle transactions, and track purchase history with ease.
6. Platform Channels
Flutter’s platform channels enable you to call platform-specific code from Dart. This feature is particularly useful for accessing native APIs that are not available in Flutter or for integrating existing native codebases into your Flutter app.
static const platform = MethodChannel(‘com.example.myapp/channel’);
Future<void> _getNativeData() async {
try {
final String result = await platform.invokeMethod(‘getNativeData’);
print(result);
} on PlatformException catch (e) {
print(“Failed to get native data: ‘${e.message}’.”);
}
}
7. Animations and Transitions
Flutter’s animation library is powerful and versatile. With widgets like AnimatedContainer
, Hero
, and FadeTransition
, you can create smooth, visually appealing transitions and animations that enhance the user experience.
AnimatedContainer(
duration: Duration(seconds: 1),
curve: Curves.easeInOut,xc
// other properties
)
8. Accessibility
Accessibility is often overlooked, but Flutter makes it easy to create accessible apps. With widgets like Semantics
and accessibility features built into the framework, you can ensure that your app is usable by everyone.
Semantics(
label: ‘Close button’,
child: IconButton(
icon: Icon(Icons.close),
onPressed: () {},
),
)
9. Flutter Plugins
The Flutter ecosystem has a rich set of plugins that extend the functionality of your app. Whether you need to integrate with a third-party service or add a specific feature, there’s likely a plugin that can help. Popular plugins include firebase_auth
, google_maps_flutter
, and sqflite
.
10. Internationalization (i18n)
Reaching a global audience is made easier with Flutter’s internationalization support. Using the intl
package, you can add multiple languages to your app and ensure that it caters to users from different regions.
import ‘package:intl/intl.dart’;
String welcomeMessage() {
return Intl.message(
‘Welcome to MyApp’,
name: ‘welcomeMessage’,
desc: ‘The welcome message for the application’,
);
}
Conclusion
Flutter is packed with features that can take your app development to the next level. By exploring and utilizing these lesser-known capabilities, you can create more efficient, user-friendly, and powerful applications. Whether you’re optimizing performance with Flutter DevTools, creating custom animations with CustomPainter
, or ensuring accessibility for all users, these secret features are sure to enhance your Flutter development journey.
Happy coding!
Join 10,000 subscribers!
Join Our subscriber’s list and trends, especially on mobile apps development.I hereby agree to receive newsletters from Mobmaxime and acknowledge company's Privacy Policy.